Welcome to today’s post.
Today I will be discussing why as a developer you should be writing clean code.
In most of my posts I have been showing you how to develop a solution to a problem using various application frameworks and development tools, and code. I will be giving you an overview of what you should do as developer to ensure that your code is clean.
In the next section I will define what clean code is by providing a check list of minimum standards.
Defining Clean Code
What is clean code?
Clean code means that your code should satisfy the following minimum standards:
Each method or function should serve one purpose
This is part of what we all the single-responsibility part of the SOLID principle. We should adhere to this principle not just for classes, but also for functions and methods.
Methods and functions that perform multiples tasks should be split into multiple methods or functions or delegated into another class.
Do not repeat the same code or logic in different places
Conditional structures and logic that achieves the same goal should be into a common shared class or helper methods. This will reduce the number of tests for our methods, increase code coverage and make the code more readable.
Making use of compiler features that allow lookup of shared references within the project allow for more effective refactoring. Use of the refactor into a method.. helper that is available in popular development environments such as Visual Studio and Visual Studio Code is quite useful.
Eliminate excessively nested structures
Methods where we use multiple nested if .. else statements can be reduced further.
Example:
Bad:
If (book.LoanBook() == BOOK_ALREADY_BOOKED)
{
If (book.CheckStatus() == BOOK_LOANED)
{
log.info($“Book {book.Name} has been loaned.”);
}
}
Else
{
log.error($”Book {book.Name} cannot be loaned.”);
}
Good:
try
{
book.LoanBook();
book.CheckStatus();
}
catch (Exception ex)
{
log.error($”Book {book.Name} cannot be loaned.”);
}
Reduce the number of function and method arguments
The greater the number of parameters in your methods or functions, the harder they are to test. The number of unit test combinations for these functions and methods will be greater.
Where possible, encapsulate the parameters into classes, with the parameters being class members.
Remove all unused variables and methods in your code
Using a Linting tool to detect code smells within the code, including checking for unused variables, unreachable code, and unused methods.
Many development IDEs such as Visual Studio Code and Visual Studio have built-in linting tools that. Other code quality analysis tools such as Resharper, SonarLint, StyleCop and Roslyn can be installed as plug-ins to give continuous feedback on code quality issues.
Ensure your variable, method and functions are named appropriately
Ensure your variable, method and functions are named appropriately, matching the intended purpose of your requirements. Use standard naming conventions when building variable, classes, and library names, using appropriate verbs and nouns in your names.
Example of a badly named variable:
int idx;
Example of a correctly named variable:
Int indexCounter;
Example of badly named class name:
public class logger1
Example of correctly named class name:
public class FileLogger
Example of badly named component name:
multiselectcomponent
Example of correctly named component name:
multi-select-component
Do not leave unnecessary comments in code
All code if named appropriately should be readable, understandable, and self-explanatory. If the code cannot be understood by another developer, then the variables within your code has not been adequately named. Code that has excessive comments will need a combination of variable renaming and refactoring to convert it to readable code
If you need to keep commented out code, there are numerous ways to save unwanted code using git stash with Git source versioning or use TFS or VSTS to shelve code. The original code can always be retrieved or viewed from the VCS history so there is no need to keep it.
Only include a comment if it serves a very compelling reason to explain a unique piece of code.
Be consistent when naming different types
You should use Camel case for variables, Pascal case for classes, Kebab case for components and URLs and Snake case for constants. This helps developers understand what category your type is in without needing to look them up.
Example of consistent variable name (Camel cased):
var productName;
Example of inconsistent variable name (Pascal cased):
var ProductName;
Example of consistent class name (Contains a noun and verb):
public class SavouryProducts;
Example of inconsistent class name (Is a verb):
public class Tasty;
Example of consistent URL (Snake casing):
http://server/any-useful-name
Example of inconsistent URL (Concatenated string):
http://server/anyusefulname
Keep your code correctly indented
Keep the indentations consistently spaced from the left and ensure that any code within a block starts on a new line, with ending left braces of a block continued onto a new line.
Clearly the following class is not easily readable:
public class Square: Shape { public int Length { get return this.width; } public int Area { get return (this.width * this.height); } }
But the same code properly indented is easily readable:
public class Square: Shape {
public int Length {
get return this.width;
}
public int Area {
get return (this.width * this.height);
}
}
In the final section, I will explain why it is just not enough for your code to just work or just pass the tests.
Is it Enough for the Code to Just Work?
Why should my code be clean?
After all I have delivered a solution that works for the customer or stakeholder!
I will explain why. The following reasons are all why you should keep your code clean:
- Your code changes are a contribution to a repository, that is used by other developers. Expecting other developers to clean up your code adds time and resources to the project that they could dedicate elsewhere.
- Adding messy code to a system adds to the technical debt of a system.
- Messy code are code smells that can if not cleaned out of a system will lead to bad habits forming for other developers.
- Unclean code is not as testable as clean code.
- Unclean code has lower test coverage than clean code. The code should be cleaned and refactored before a test engineer can write unit tests for the code.
The above discussion does not cover every possible situation for clean code rectification. To be proficient at keeping your code base clean requires continual practice as each code base throws up different challenges.
That is all for today’s post.
I hope you found it useful and informative.
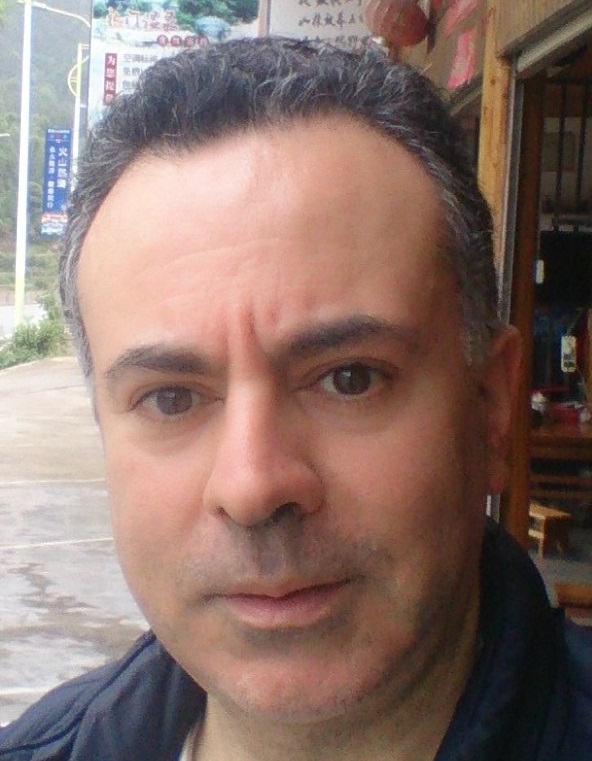
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.