Welcome to today’s post.
In this post I will be explaining what a Blazor web application is, how it fits into C# and .NET with the Razor components, how the ASP.NET Core Blazor server architecture interacts with the client browser DOM, and how to create a basic application from Visual Studio 2022. I will also give an overview of the different types of Blazor web applications and when they can be used when building a web application.
In the first section, I will explain what the Blazor web development framework is.
What is Blazor?
Blazor is a web development framework built on top of ASP.NET Core, that uses the development language C#, HTML markup and CSS. One of the key features of the Blazor framework are:
- reusability of components that can be combined with other components to produce more complex interface components.
- developing web interfaces in the C# language, which allows developers who may have been accustomed to using C# in different types of .NET applications, such as web clients, windows forms clients, and server components. Being able to leverage existing C# scripting language skills allows developers to transition on develop front-end clients with both C# and HTML in the same source code file.
- reusing the same code in both the server and client build of the web application.
If you have previously implemented web applications using the ASP.NET MVC (or ASP.NET Core) framework with the Razor UI scripting language, then you will be familiar with how you can combine HTML scripting with conditional logic with variables, objects, and view model instances. The diagram below shows the component and language architecture of how applications in Blazor are structured and the development languages that are used in each type of Blazor script or component source file:
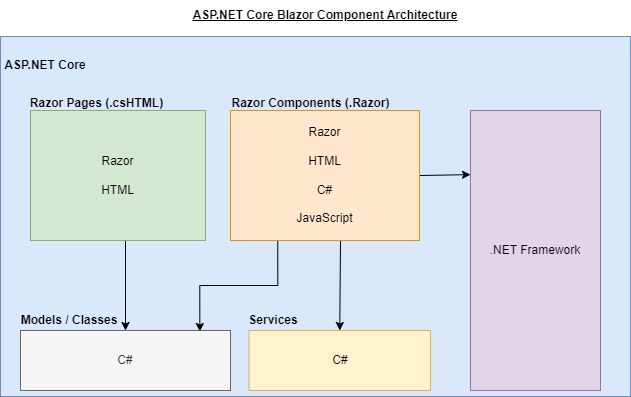
I will show later how the above languages are used within Blazor Razor components as they are very similar to how you would have used the same Razor scripting language in ASP.NET MVC and ASP.NET Core MVC applications. The main difference between the Blazor Razor HTML pages and Blazor Razor Components is the additional capability of embedding C# and JavaScript into the component file’s code section.
With traditional HTML and JavaScript, we would need to enclose any JavaScript code within a <script>..</script> block. With the Razor UI scripting syntax, we can use the @ to prefix any variable, object instance, command, or C# code block. An example of a reference to an object instance is below with a property of the current DateTime object instance:
<p>@DateTime.Now</p>
Another example is when referencing an assigned variable created within the Razor script:
@{
var message = "The best is yet to come! ";
}
<p>@message</p>
Applying conditional logic is when we use the @if command with a conditional expression, then include markup within an HTML block:
@if (file_size > 10000000)
{
<p>The maximum file size has been exceeded.</p>
}
With Blazor server apps we use event handlers from user interface elements to C# methods using the @onxxx directive, like this for a click event:
@onclick=”someMethod”
With traditional JavaScript code, the event handler for a click event would be declared as shown:
onclick=”someMethod()”
In the next section, I will explain the different types of Blazor applications.
The Types of Blazor Applications
There are two different types of Blazor applications, and they coincide with the hosting model for the web application.
Blazor Server Apps
The first hosting model is the Blazor Server App. With this model, the application is hosted and run on the server, with the application running under ASP.NET Core. Any user interface interactions that include events from the browser are sent to the server, which then applies any updates to interface components to the client DOM elements. All user interface updates are handled through a SignalR web sockets connection.
A general architecture of how Blazor Server application requests, connections, and browser events interact between the server and browser client is shown below:
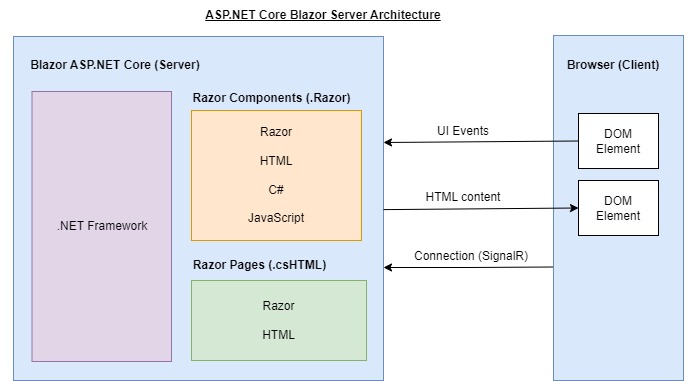
Blazor WebAssembly Apps
The next hosting model is the Blazor WebAssembly App. With this model, the application .NET code is generated on the web server, then downloaded and run on the client browser. The client effectively runs offline with all interactions occurring within the browser client. There are no server dependencies such as databases on the client side, therefore, any server calls are executed through HTTP REST calls.
More details on the WebAssembly will be in a future post.
In the next section I will explain how the Blazor starter application is created and explain the individual interface components that it consists of.
Creating a Starter Blazor Server Web App
To create a basic Blazor Server web application we can use the Visual Studio 2022 project creation template. Selecting the application type Blazor and the C# language gives us either the Blazor Server App or the Blazor WebAssembly App. With each type we can choose either a minimal starter app or an empty app.
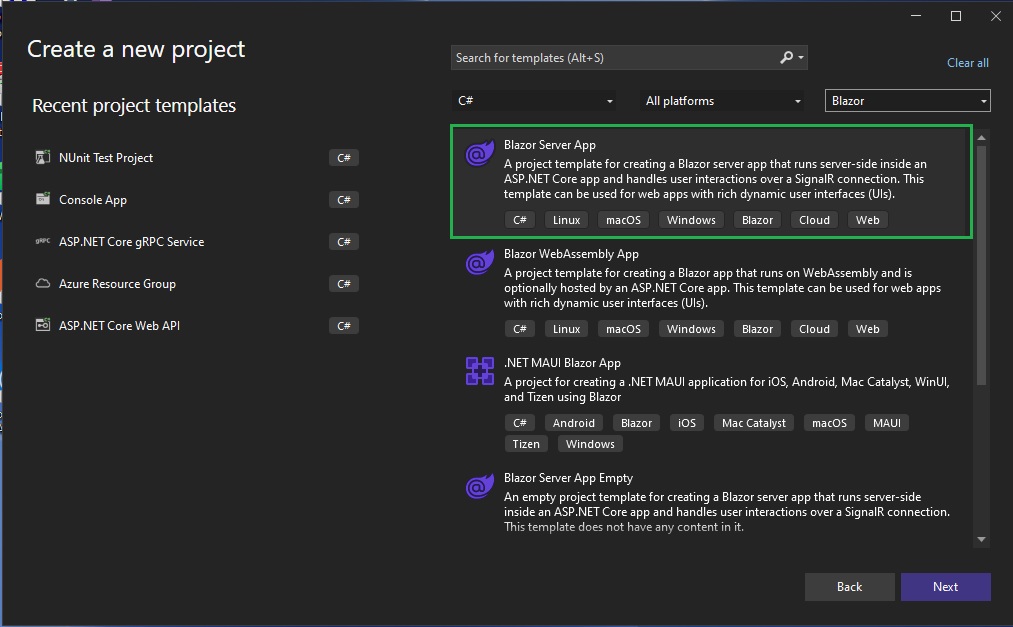
After the project is created, the Solution Explorer will show the application solution structure of the Blazor Server App. This is shown below:
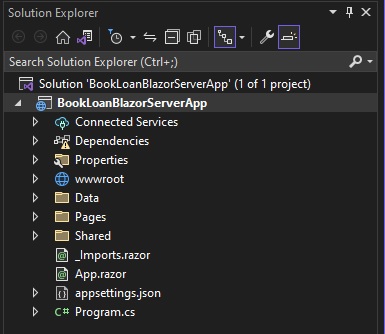
Notice there is a Pages folder that contains razor component pages and cshtml HTML markup pages. Each razor component has an associated CSS stylesheet file.
There is also an App.razor page that bootstraps the application.
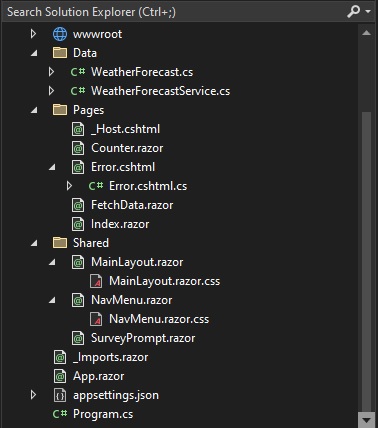
After the application is built and run, you will see the following web application landing page, which contains a welcome page and a side navigation panel with some links to sample pages:
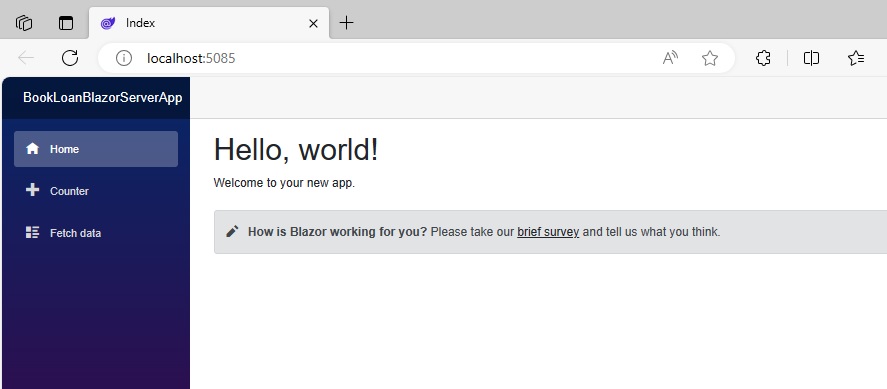
When the Counter link in the side panel is clicked the page for the counter demo shows which increments the count in a label for each time we click on the blue button:
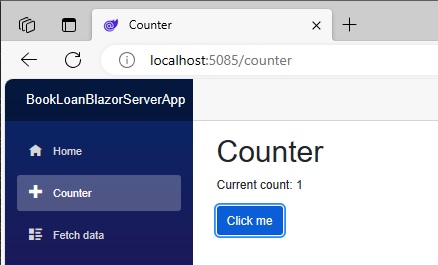
The Counter.razor component code is shown below:
@page "/counter"
<PageTitle>Counter</PageTitle>
<h1>Counter</h1>
<p role="status">Current count: @currentCount</p>
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
}
The code for the razor component is constructed from a hybrid of HTML markup and C# code within its own code block.
To define the routing for the razor component, we use the @page directive. The page routing for the above component is below:
@page "/counter"
What this tell us is that the routing for the razor component requires the following URL to open the counter page (the port will differ in your own development environment):
http://localhost:5085/counter
In the component, the section of code that defines the click action for the counter button is within the @code { … } block, which contains a method, IncrementCount(), which increments the value of the private variable, currentCount.
The C# code is shown below:
@code {
private int currentCount = 0;
private void IncrementCount()
{
currentCount++;
}
}
The HTML script for the button then references the C# method, IncrementCount() using the @onclick event handler. Recall that with JavaScript web applications, we would reference a JavaScript method from an interface element click event using the onclick JavaScript event handler, and would be referenced with onclick=”incrementCount()”.
The C# onclick event handler within the HTML button definition is declared as shown:
<button class="btn btn-primary" @onclick="IncrementCount">Click me</button>
When we click on the Fetch data menu item, we see a page containing a grid with populated weather data:
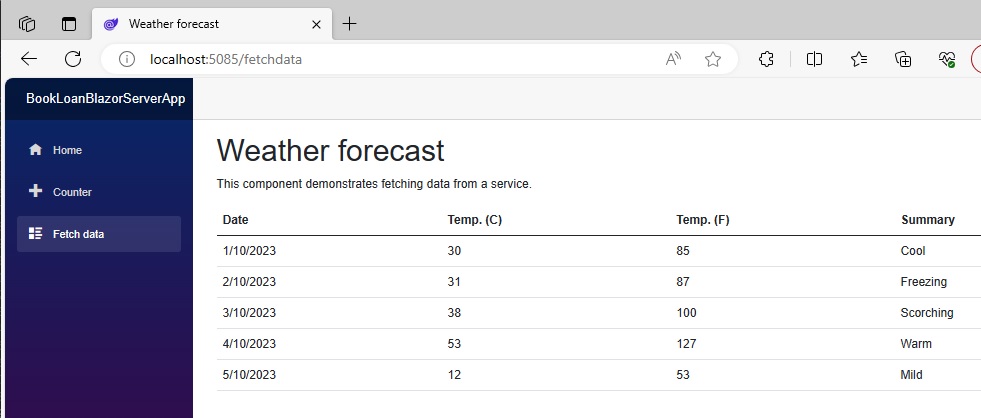
The razor component FetchData.razor for the above page is shown below:
@page "/fetchdata"
@using BookLoanBlazorServerApp.Data
@inject WeatherForecastService ForecastService
<PageTitle>Weather forecast</PageTitle>
<h1>Weather forecast</h1>
<p>This component demonstrates fetching data from a service.</p>
@if (forecasts == null)
{
<p><em>Loading...</em></p>
}
else
{
<table class="table">
<thead>
<tr>
<th>Date</th>
<th>Temp. (C)</th>
<th>Temp. (F)</th>
<th>Summary</th>
</tr>
</thead>
<tbody>
@foreach (var forecast in forecasts)
{
<tr>
<td>@forecast.Date.ToShortDateString()</td>
<td>@forecast.TemperatureC</td>
<td>@forecast.TemperatureF</td>
<td>@forecast.Summary</td>
</tr>
}
</tbody>
</table>
}
@code {
private WeatherForecast[]? forecasts;
protected override async Task OnInitializedAsync()
{
forecasts = await ForecastService.GetForecastAsync(DateOnly.FromDateTime(DateTime.Now));
}
}
The first three lines:
@page "/fetchdata"
@using BookLoanBlazorServerApp.Data
@inject WeatherForecastService ForecastService
Are self-explanatory, with the page routing for the fetch data page being:
http://localhost:5085/fetchdata
The @using directive declares the data source for the page.
The @inject is used to inject an interface to a concrete implementation, WeatherForecastService using dependency injection. I will explain dependency injection with Blazor server apps in a later post.
The rest of the HTML markup combines a Razor conditional statement block:
@if (forecasts == null)
{
…
}
else
{
…
}
with a loop in the else block that retrieves the data from the injected object instance for ForecastService from:
forecasts = await ForecastService.GetForecastAsync(DateOnly.FromDateTime(DateTime.Now));
which references the asynchronous method GetForecastAsync() within the OnInitializedAsync() method within the @code { … } block.
Properties within each retrieved temperature object instance are displayed within each cell column to properties as shown:
<td>@forecast.Date.ToShortDateString()</td>
<td>@forecast.TemperatureC</td>
…
Whereas within an ASP.NET Core MVC web Razor cshtml page, we would reference properties of an instance of a Model bound object, in a Blazor Server web app we would reference properties of an instance of a razor component variable or object.
The above overview and discussion has given you some idea what a Blazor server app is and given you an overview on the similarities between the implementation of an ASP.NET Core MVC web application and an ASP.NET Core Blazor web application. We have also seen the similarities and differences with how Blazor server apps and traditional JavaScript web apps reference scripts and code with event handlers.
In future posts I will explain in more details how to other features of Blazor Server applications including event handling, component reuse, and integration of external data sources with Blazor Server applications using Entity Framework Core.
That is all for today’s post.
I hope you have found this post useful and informative.
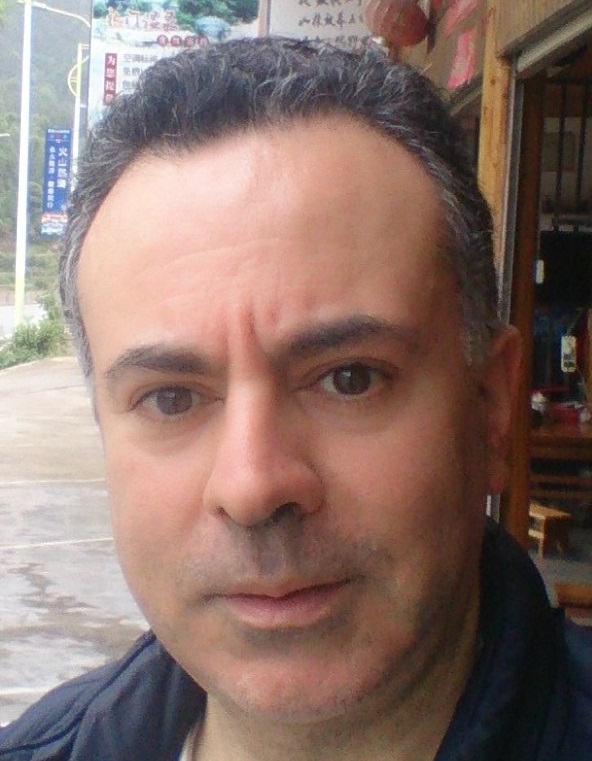
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.