Welcome to today’s post.
In today’s post I will show how to validate a single-selection drop-down list field control within an ASP.NET Core Blazor server web application.
Validations of selection controls is normally combined within other controls contained within the same data entry form within an application. The simplest controls to validate would be the basic text edit controls. Text edit controls that contain validations of the content of the text, such as regular expression patterns are next in terms of complexity. With drop-down lists, the validation is confined to a finite collection of values that the user is constrained to choose from.
I will explain the different types of single selection drop-down controls, then show how to implement the Razor markup and code for a drop-down within a Blazor .NET Core application.
Types of Single Selection Drop Down Controls
There are a two main types of single-selection drop-down selection controls that we can use for data entry:
- Drop down list control with the available options being mandatory.
- Drop down list control with the available options being optional.
The first type forces the user to make a choice. In the absence of a choice, the form validation state is invalid.
The second type gives the user an additional option, such as a blank space choice or a default such as “N/A” or “None”, which sets the corresponding data value to a blank string, a zero, or a null in the processed form data.
In a previous post, I showed how to validate from controls within an ASP.NET Core Blazor server web application. In that post, I showed how to validate Blazor input and numeric field controls. One type of control that I did not mention was a basic drop-down field control.
In terms of HTML web applications, a drop-down control can be presented within a <select> tag that contain <option> tags that represent the selection choices. Below is the basic HTML for a single-selection drop-down control:
<div class="form-field">
<label>Genre:</label>
<div>
<select>
<option value="">Select genre ...</option>
<option value="Fiction">Fiction</option>
<option value="Non-Fiction">Non-Fiction</option>
<option value="Educational">Educational</option>
<option value="Childrens">Childrens</option>
<option value="Family">Family</option>
<option value="Fantasy">Fantasy</option>
<option value="Finance">Finance</option>
<option value="Cooking">Cooking</option>
<option value="Fantasy">Fantasy</option>
<option value="Technology">Technology</option>
<option value="Fantasy">Fantasy</option>
<option value="Gardening">Gardening</option>
</select>
</div>
</div>
In the next section, I will show how drop-down lists are defined with Blazor application forms.
Drop Down List Controls in Blazor Application Forms
With ASP.NET Core Blazor Server Razor forms, the drop-down lists are declared within <InputSelect> tags that are bound to a field value within a Model. Below is an example of a Blazor drop-down field:
<div class="form-field">
<label>Genre:</label>
<div>
<InputSelect @bind-Value="editbook.Genre">
<option value="">Select genre ...</option>
<option value="Fiction">Fiction</option>
<option value="Non-Fiction">Non-Fiction</option>
<option value="Educational">Educational</option>
<option value="Childrens">Childrens</option>
<option value="Family">Family</option>
<option value="Fantasy">Fantasy</option>
<option value="Finance">Finance</option>
<option value="Cooking">Cooking</option>
<option value="Fantasy">Fantasy</option>
<option value="Technology">Technology</option>
<option value="Fantasy">Fantasy</option>
<option value="Gardening">Gardening</option>
</InputSelect>
</div>
</div>
The above HTML Razor declaration has all the selection options hard coded into the HTML markup. What if the options were from a data source or a text file where the records changed, and the selectable options differed? In this case, we could read the options from a list or data source within the model, or an enumerated reference type.
Below is our original model class that we used within the first version of our validation form:
using System.ComponentModel.DataAnnotations;
namespace BookLoanBlazorServerApp.Models
{
[Serializable]
public class BookViewModel
{
public int ID { get; set; }
[Required]
public string? Title { get; set; }
[Required]
public string? Author { get; set; }
public int YearPublished { get; set; }
[Required]
public string? Genre { get; set; }
public string? Edition { get; set; }
public string? ISBN { get; set; }
[Required]
public string? Location { get; set; }
[Required]
public string? MediaType { get; set; }
public DateTime DateCreated { get; set; }
public DateTime DateUpdated { get; set; }
public BookViewModel() { }
}
}
As you can see, the Genre and MediaType fields would be ideal candidates as drop-down selectable fields. Currently they were declared as strings with a [Required] validation annotation.
To expose the Genre and MediaType fields to the Razor component, we would need to create a different Model, BookEditModel, that is derived from the BookViewModel class.
Below is the declaration of the BookEditModel, with the Genres and MediaTypes properties of type Dictionary<int, string> with public visibility:
namespace BookLoanBlazorServerApp.Models
{
[Serializable]
public class BookEditModel: BookViewModel
{
Dictionary<int, string> _media_types;
Dictionary<int, string> _genres;
public Dictionary<int, string> Genres
{
get { return _genres; }
}
public Dictionary<int, string> MediaTypes
{
get { return _media_types; }
}
public BookEditModel(BookViewModel vm)
{
ID = vm.ID;
ISBN = vm.ISBN;
Author = vm.Author;
Edition = vm.Edition;
Genre = vm.Genre;
Location = vm.Location;
MediaType = vm.MediaType;
Title = vm.Title;
YearPublished = vm.YearPublished;
DateCreated = vm.DateCreated;
DateUpdated = vm.DateUpdated;
CreateLookups();
}
public BookEditModel()
{
CreateLookups();
}
private void CreateLookups()
{
this._genres = new Dictionary<int, string>()
{
{ 1, "Fiction" },
{ 2, "Non-Fiction" },
{ 3, "Educational" },
{ 4, "Childrens" },
{ 5, "Family" },
{ 6, "Fantasy" },
{ 7, "Finance" },
{ 8, "Cooking" },
{ 9, "Technology" },
{ 10, "Gardening" }
};
this._media_types = new Dictionary<int, string>()
{
{ 1, "Book" },
{ 2, "DVD" },
{ 3, "CD" },
{ 4, "Magazine" }
};
}
}
}
In the next section, we will see how to define the drop-down lists within Blazor application components.
Creating Drop-Down Lists in Blazor Forms
In the Razor component HTML markup, we render the drop-down lists by replacing the Razor <InputText> element controls with a an <InputSelect>…</InputSelect> element that contain selectable options with <option value=…></option> elements.
Below is a declaration of an input text element for the Genre field:
<div class="form-field">
<label>Genre:</label>
<div>
<InputText @bind-Value=editbook.Genre></InputText>
</div>
</div>
Which we replace with an <InputSelect> element with selectable options:
<div class="form-field">
<label>Genre:</label>
<div>
<InputSelect @bind-Value="editbook.Genre">
<option value="">Select genre ...</option>
@foreach (var genres in @editbook.Genres)
{
<option value="@genres.Value.ToString()">@genres.Value.ToString()</option>
}
</InputSelect>
</div>
</div>
Instead of hard coding the selectable options, we iterate through them from the models Genres property as shown:
@foreach (var genres in @editbook.Genres)
{
<option value="@genres.Value.ToString()">@genres.Value.ToString()</option>
}
Referencing each value within the property is done with the Value property.
After we have declared the edit context, we have the following code in the initializer to create an instance of the BookEditModel model, sub-classing it from the view model:
private BookViewModel? book;
private BookEditModel? editbook;
...
protected override async Task OnInitializedAsync()
{
// Retrieve book record
book = await LibraryBookService.GetBook(ID);
// Convert object BookViewModel into object of type BookEditModel
editbook = new BookEditModel(book);
// Establish edit context of type BookEditModel
editContext = new(editbook!);
editContext.OnFieldChanged += HandleFieldChanged;
}
The rest of the C# code is almost the same as for the code I showed in the post, where I showed how to use Entity Framework in ASP.NET Core Blazor applications, except we use the editbook instead of the book object in the UpdateBook() method as shown:
// Update entered book properties.
private async void UpdateBook()
{
var result = await LibraryBookService.UpdateBook(ID, editbook! as BookViewModel);
if (result != null)
message = "Record has been successfully updated!";
else
message = "Unable to update record.";
await JS.InvokeVoidAsync("alert", message);
}
The HTML and Razor script then references editbook for each remaining control as we did for the <InputSelect> drop down selection control element:
<div class="form-field">
<label>Title:</label>
<div>
<InputText @bind-Value=editbook!.Title required></InputText>
</div>
</div>
When we run the application and select the record edit, the drop-down controls will display as shown (provided we have added Razor markup for the media type control as well):
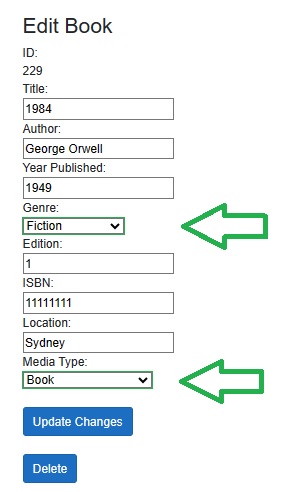
Clicking on the first drop down list we can see the same entries that we had defined in the model property Genres:
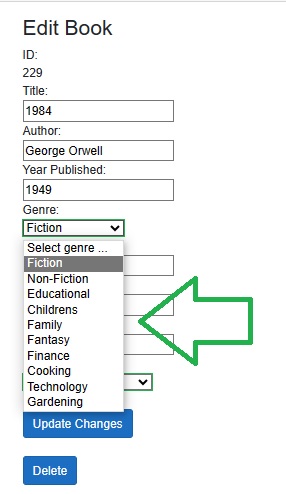
Similarly, the MediaTypes property is rendered in the media type drop-down list as shown:
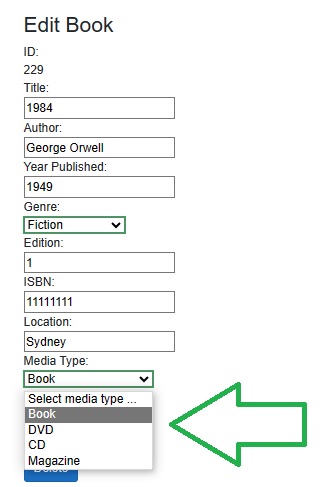
To test validation for any of the drop-down list controls, select the option: Select … as shown below:
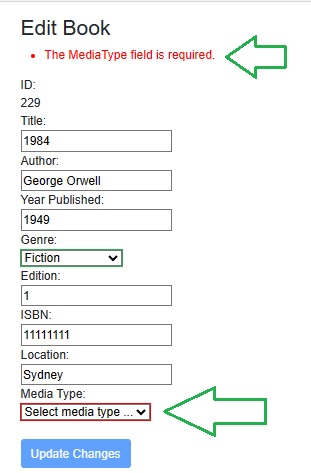
You will immediately notice the following changes in the interface:
- A red border surrounds the drop-down control element.
- In the validation summary area of the form, you will see the error message display:
The MediaType field is required.
- The Update Changes button will be disabled.
The above overview has shown us how to validate drop-down lists in an ASP.NET Core Blazor form component.
We saw how to complete the following tasks during the implementation of a validated drop-down list within a Blazor Form:
- Defining the choices within a suitable list data structure or model.
- Define Razor HTML markup to display any invalid controls in validation summary.
- User feedback to highlight any fields where there is an invalid selection in the drop-down list.
- Preventing form submission for an invalid drop-down list selection.
That is all for today’s post.
I hope that you have found this post useful and informative.
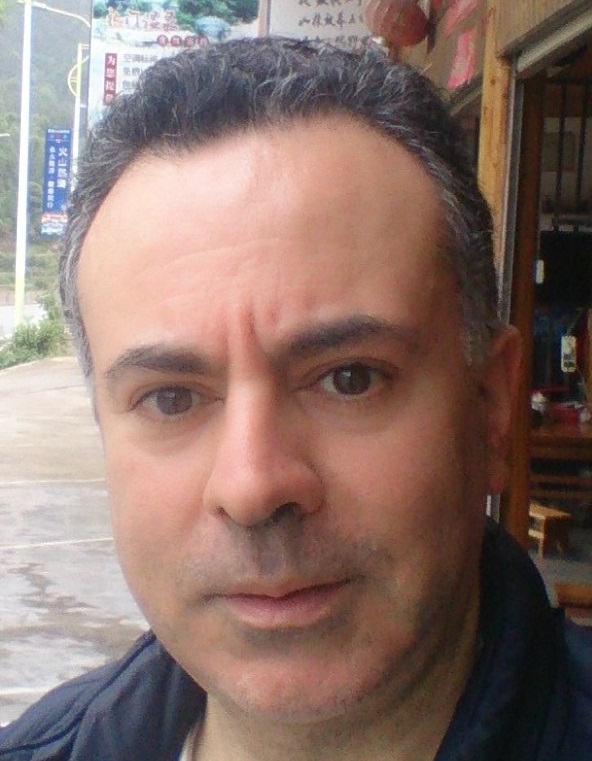
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.