Welcome to today’s post.
In this post I will be showing how to retrieve user roles from JWT tokens using .NET Core.
In a previous post I showed how to embed user roles into a JWT token.
You would be asking, what is the purpose behind retrieving a role from within a JWT token? The most common reason we have for retrieving roles from a JWT token is to use the role to control authorization of resources within the application.
Before we could retrieve the role, we had to determine the validity of the JWT token after we had signed into the application. The validity of the token meant that we had established authentication within the application. Once we were authenticated, we could access the application resources. With some applications, we might have finer granularity in the way we control access to resources within our application. These can include access to a subset of methods within each service or business rules that depend on the current role of the signed in user.
The most common use of roles is to implement authorization within applications or web API services.
In another previous post I showed how to obtain and persist a JWT token into a web application or web API cookie.
From the token that is stored within the request cookie, we convert the token, which is a bearer token to a ClaimsPrincipal object, then search through the claims within the Claims property of the ClaimsPrincipal object and filter by the Type property of each claim object, matching it against types matching ClaimsTypes.Role.
Below is our method that is used to determine if the application’s current user is a member of the given role:
public async Task<bool> IsCurrentUserInRole(string role)
{
bool rslt = false;
string bearerToken = _context.Request.Cookies["token"];
ClaimsPrincipal claimsPrincipal =
_tokenManager.GetPrincipal(bearerToken);
foreach (Claim claim in claimsPrincipal.Claims)
{
if (claim.Type == ClaimTypes.Role)
{
if (claim.Value == role)
{
rslt = true;
break;
}
}
}
return rslt;
}
The above would work if we enabled cookies as an authentication method. If we enabled token-based authentication, then the method of retrieval of our token value would be Request.Headers.HeaderAuthorization.
Further details of our token generation implementation can be seen in a previous post. If our token fails to validate the result returned will be a null.
During debugging, the ClaimsPrincipal obtained from the token manager contains a number of claims of which we can obtain values from the role claims:
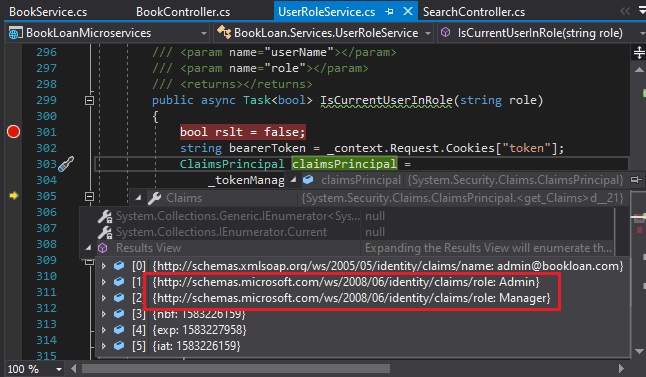
The above method can be called from any methods within our application or exposed as an API method. Below we show an example of use from Razor UI cshtml:
@if (await UserRoleService.IsCurrentUserInRole("Admin"))
{
{
<a asp-area="" asp-controller="Book" asp-action="Delete" asp-route-id="@Html.ValueFor(model => model.BookViewModel.ID)"> | Delete Book</a>
}
}
The above overview has shown you how to retrieve roles from a JWT bearer token when cookies are enabled as the authentication method. It should prove to be one invaluable tool to utilise for application security authorization purposes.
That’s all for today’s post.
I hope this post has been useful and informative.
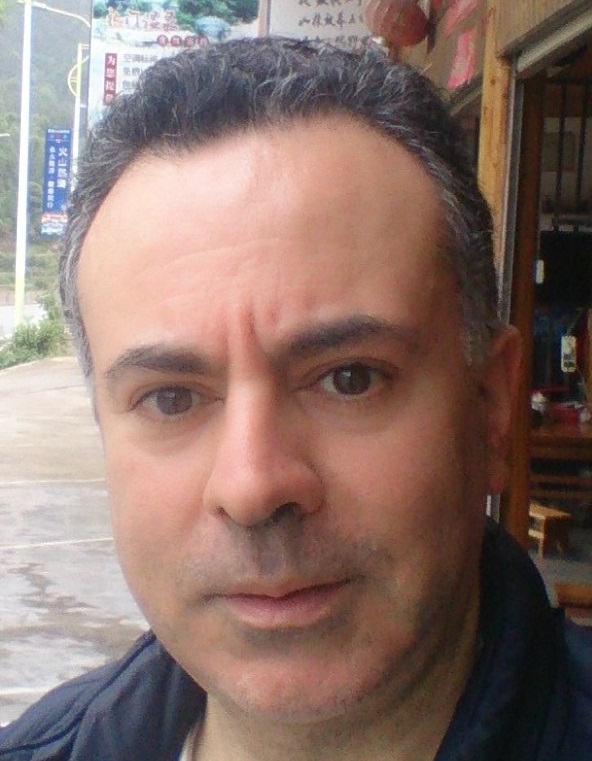
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.