Welcome to today’s post.
Today I will show how to debug unit tests for Angular applications running under the Karma Test Runner.
Unit tests within Angular applications are defined within test script source files that have the naming pattern:
[component-name].component.spec.ts
By default, the Angular development framework within Visual Studio Code allows us to manually run unit tests during the development cycle. One limitation of this is that we cannot re-run the tests automatically after a unit test file has changed. In addition, the unit test result outputs are unformatted in the terminal output windows. A dedicated test runner provides us with some additional configurations that allow test runs to be automated with more user-friendly results.
What is the Karma Test Runner?
The Karma Test Runner is a framework for allowing developers to continuously run tests that are written using a BDD script such as Jasmine. Unlike other unit test frameworks that we are accustomed to, this test framework allows the developer to receive feedback as they are writing the tests. In Angular we have a similar development environment, where changes to code are rebuilt continuously using Web Pack. The Karma test runner runs as a web server and executes tests on source code that is connected to browsers that are connected to the test runner.
The karma.config.js file is where Karma is configured. There are a few useful configuration keys to be aware of:
Base search path
The base path is where Karma checks for changes to source files in your project. The path in Angular is relative to where configuration file is located. The default is blank as shown in the example below:
basePath: ''
Auto watch
The auto watch is a flag that indicates that Karma will check for file changes if true, or not check for file changes when false. The default is set to true as shown:
autoWatch: true
Single run
The single run flag indicates if Karma will run one or more tests for each test runner session that is started. The default is false as shown:
singleRun: false
Restart on file change
The restart on file change if set to true will restart a running test if there are any file changes detected, otherwise it will let the currently running test to end before restarting. The default is true as shown:
restartOnFileChange: true
Running Unit Tests with the Karma Test Runner
Before running unit tests within Angular, ensure that you have at least one unit test implemented in your application.
For example, I have a unit test implemented in a component as shown:
import { async, ComponentFixture, TestBed } from '@angular/core/testing';
import { MaterialModule } from '../material/material.module';
import { BooksComponent } from './books.component';
import { TestApiService } from '../services/test-api.service';
import { ApiService } from '../services/api.service';
import { Router } from '@angular/router';
import { getBooks } from '../services/test/test-books';
const books = getBooks();
describe('BooksComponent', () => {
let component: BooksComponent;
let fixture: ComponentFixture<BooksComponent>;
let mockRouter = {
navigate: jasmine.createSpy('navigate')
};
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [ BooksComponent ],
imports: [ MaterialModule ],
providers: [
{ provide: ApiService, useClass: TestApiService },
{ provide: Router, useValue: mockRouter }
]
})
.compileComponents();
}));
beforeEach(() => {
fixture = TestBed.createComponent(BooksComponent);
component = fixture.componentInstance;
let books = component.books;
if (books)
console.log("book length=" + books.length);
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
it('should display books', () => {
expect(books.length).toBeGreaterThan(0);
});
});
Run unit tests for Angular with the following command:
ng test
When the tests start running you will see the Karma test runner launch in the Chrome browser window.
Debugging Unit Tests with the Karma Test Runner
There is also a Debug button shown in the top right corner.
Once you have clicked on this you will be taken to the next screen, your tests will be in debug mode.
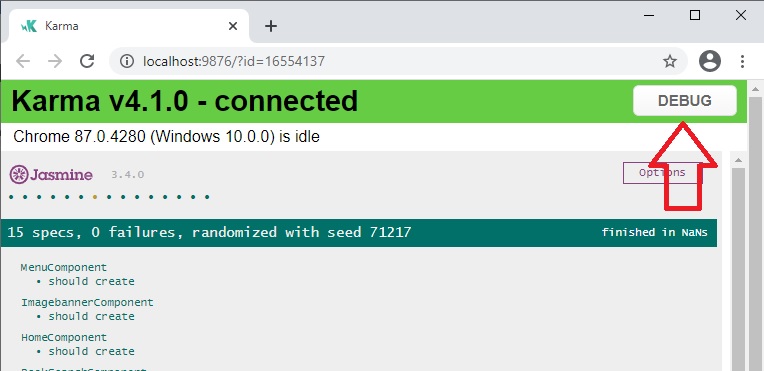
Now open the Chrome Development Tools.
Select the Sources tab.
When presented with the source folder, locate the _karma_webpack_ folder, then expand the folders until you locate the test source file that you wish to debug.
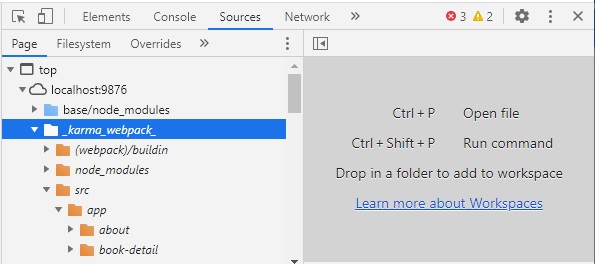
The file will have an extension, .spec.ts.
Select the file to open it in the source pane. Add the breakpoint to the source line.
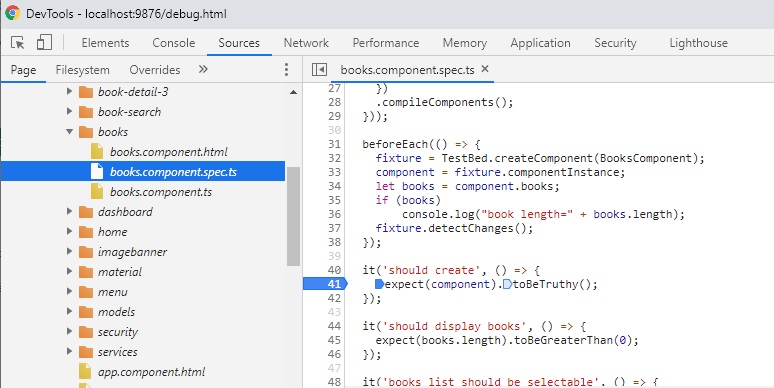
Refresh the Karma test runner browser as shown:
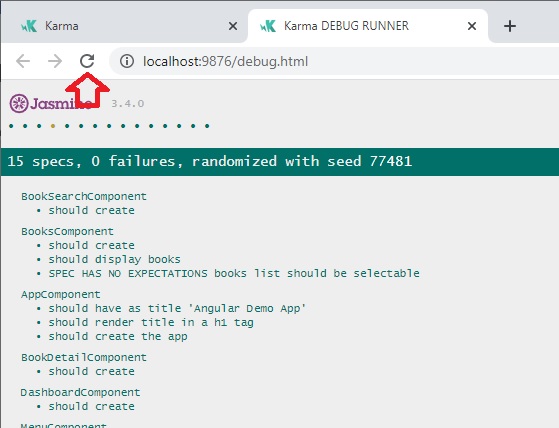
The component unit tests will start running from the beginning. Once the component of the source containing the break point starts running, the debugger will pause at the breakpoint in the source within the dev tools.
We can then use the dev tools to inspect and watch the variables local to the test suite.
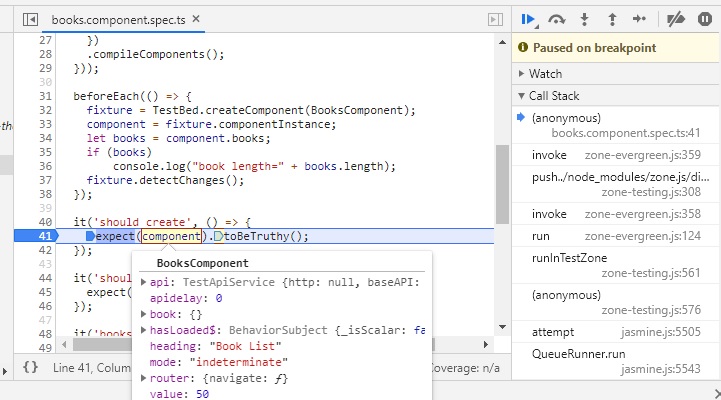
Once debugging is completed, continue the debugger and the test runner will complete running all tests.
You have seen how useful the Karma Test Runner is in running and reporting our end-to-end Angular tests with higher productivity feedback and no manual reconfiguring or rebuilding during the development phase empowering developers with the capability to be both a developer and tester.
That’s all for today.
I hope this has been useful and informative post for helping diagnose and troubleshooting issues during development of Jasmine unit testing of Angular applications.
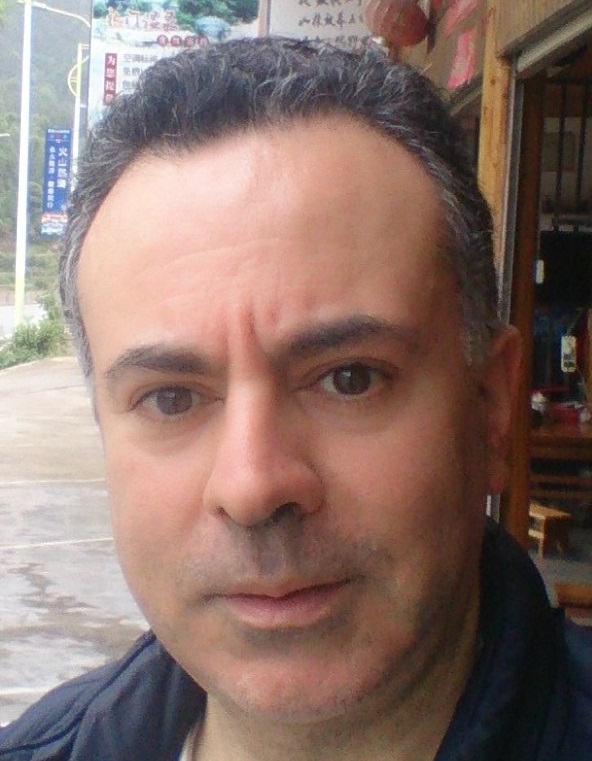
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.