Welcome to today’s post!
In this post I will discuss how to use notifications to give responsive and user-friendly notifications to the end-user of your Angular application.
The most common notification we are accustomed to are text notifications that appear on your form after a completed task. The notifications can be in a color such as red indicating an error, or in green which indicate a successful operation.
When our Angular front-end communicates with a backend service, the use of notifications from resulting responses is essential, as few end-users understand server responses that only developers and trained technical support staff can interpret. As developers it is our requirement to translate such responses into meaningful visual notifications for end users.
From the end-user view, the use of feedback is important when an action is completed. The user wants to be reassured that they are using the system in the intended way. In addition, when an action is still in progress, there still needs to be feedback in the form of progress.
Feedback with the SnackBar Component
There are several components in the Angular Material library that can provide end-user feedback, such as progress bars, batches, and toasters.
I will introduce the use of a component from the Angular Material library. This component is the SnackBar component.
The SnackBar component is used to display a toaster-like visual notification to the end user.
In the next section, I will go over an example where notifications are sent from an external web API and processed in a component that contains a SnackBar component.
Notifications from an Authentication Service
I will go through an example where the user logs into the application from a login form and returns a meaningful message to the login form. The login form then processes the message and posts a visual notification to the end user. In our authentication service we have the following artifacts:
- A Subject multicast observable to hold and emit the login results to the login form. We define the loginResponse to store this value. Initially there will be no initial values.
public loginResponse: Subject<string> = new Subject<string>();
- A login method to call an authentication API, then process the resulting response message. There will either be a successful message (such as “login successful”), or an error message (such as “invalid password” or “login name is invalid”). The message will then be emitted as an observable through the authentication service’s loginResponse variable.
login(userName: string, password: string) {
var config = {
headers: new HttpHeaders({
'Content-Type': 'application/json',
})
};
this.http.post<any>('http://localhost/BookLoan.Identity.API/api/Users/Authenticate', { "UserName": userName, "Password": password }, config)
.subscribe(
res => {
this.loginResponse.next("login success");
},
error => {
this.loginResponse.next(error);
},
()=> {
}
);
}
In our login form component, the login() method will subscribe to the loginResponse observable. When the result is emitted from the authentication service, it will be processed within the subscriber and forwarded to the SnackBar with the login response:
login(username: string, password: string): void {
this.auth.login(username, password);
this.auth.loginResponse.subscribe(r =>
{
this.openSnackBar("Login", r);
});
}
Configuration of SnackBar Notifications in a Component
The SnackBar is defined within our login component as follows:
- Import declaration:
import { MatSnackBar } from '@angular/material';
- Inject the MatSnackBar component from the constructor:
constructor(private auth: AuthService, private snackBar: MatSnackBar,
private fb: FormBuilder) {
this.form = fb.group({
email: ['', Validators.required],
password: ['', Validators.required]
})
}
- Create a method to display the snack bar:
openSnackBar(message: string, action: string) {
this.snackBar.open(message, action, {
duration: 3000
});
}
The snack bar requires a duration in milliseconds which is used to keep the message displayed to the end-user. The message will then disappear after the duration elapses. In the above case, I have set the duration to 3 seconds.
To standardize our snack bar a color scheme used by our application, we can use a CSS style to define the background color within styles.scss:
.blue-snackbar {
background: cadetblue;
white-space: pre-wrap;
}
Then we set the panelClass property for our snack bar component as shown:
openSnackBar(message: string, action: string) {
this.snackBar.open(message, action, {
duration: 3000,
panelClass: ['blue-snackbar']
});
}
When we run the application and attempt an invalid login, we get the following notification:
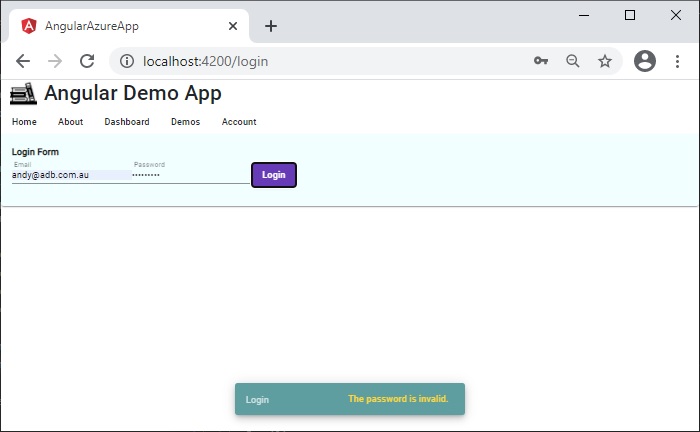
When we login successfully, the notification message displays, and we will get routed to the home page:
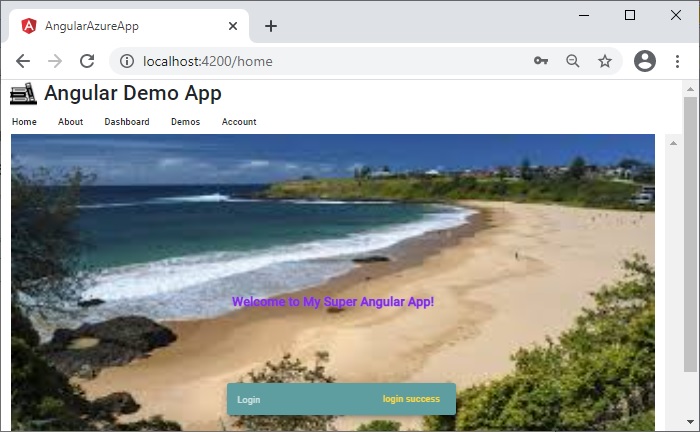
Notice that the notification will persist for a short time after we route to another page and will disappear after the duration elapses.
I have showed a useful way to utilize the Angular Material snack bar component. We could use the same notification for the following situations:
- File upload or download completions.
- Data entry and CRUD operations on a form.
- Importing to or form an external file.
That is all for today’s post.
I hope you have found this post useful and informative.
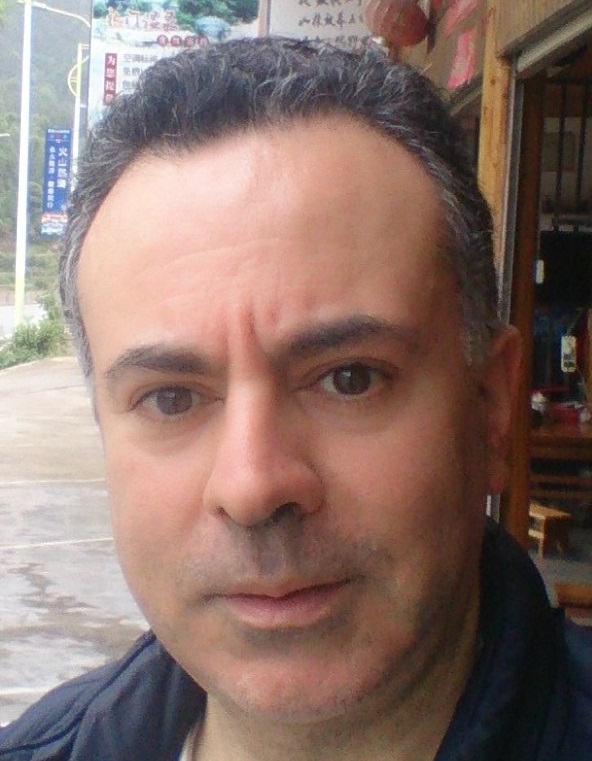
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.