Welcome to today’s post.
In today’s post I will be explaining what the Hot Reload feature is from the Visual Studio development environment.
The Hot Reload feature has been available in Visual Studio since Visual Studio 2019 and still available in Visual Studio 2022. It allows the developer who is debugging their application to reload modified code or markup scripts in IDE source code into a running instance of the running application.
Benefit of using the Hot Reload Feature
The Hot Reload feature allows the source code changes to be applied within a part of the memory of the running instance of the application. This then allows the developer to execute that same piece of code from another part of the application while the application instance is running. The changes are then visibly reflected when dependent views are displayed.
The benefit behind this feature is to allow developers to not need to restart their application or stop and start their application every time a change is made to either the code-behind of the interface or to the markup script of a visible page view within the application. Every time the application is started after a code change has been made, the application is recompiled, rebuilt and run. This is very time-consuming, especially when you have other resources running on your machine.
I will go though an example where it can be used for changes to both code-behind and markup script while an application runs during debug mode. I will go through the Hot Reload settings and how they are used during the running of the application.
Using Hot Reload in a .NET Application
The application I am running is a very rudimentary one that has a basic landing page and a link to a like of books as shown:
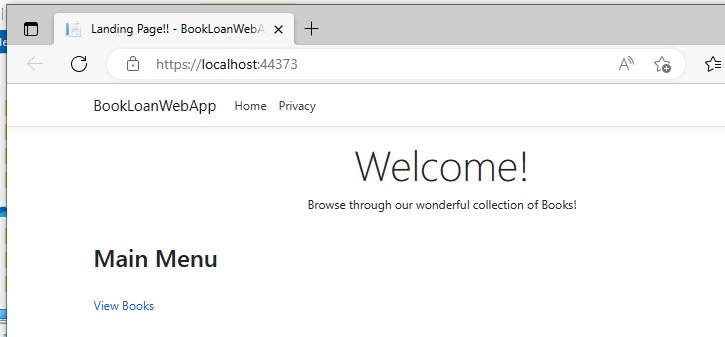
The experiment I will go through will cover changes to the landing page and to the book listing page on both code and view markup.
The application will use ASP.NET Core MVC with controllers and Razor markup pages (CSHTML).
I will go through the important parts of the source to show the sections of code and markup that will be affected during the hot reloads.
The Index page CSHTML markup is shown below:
Index Page
@model IndexModel;
@{
ViewData["Title"] = "Landing Page!!";
}
<div class="text-center">
<h1 class="display-4">@Model.WelcomeMessage</h1>
<p>Browse through our wonderful collection of Books!</p>
</div>
<br />
<h2>Main Menu</h2>
<br />
<div>
@Html.ActionLink("View Books", "List", "Book", null, new { id="viewBookLink" })
</div>
The Index page model is shown below:
Index Page Model
namespace BookLoanWebApp.Models
{
public class IndexModel
{
public string WelcomeMessage { get; set; }
public string HeadingMessage { get; set; }
}
}
Below is the section of code from the Index action within the Home controller that creates an instance of the Index view:
Index in Home Controller
public IActionResult Index()
{
IndexModel indexModel = new IndexModel() {
WelcomeMessage = "Welcome!",
HeadingMessage = "Browse through our wonderful collection of Books!"
};
return View(indexModel);
}
The Book List page CSHTML markup is shown below:
Book List Page
@model BookLoanWebApp.Models.BookListViewModel;
@{
ViewData["Title"] = "Book List";
}
<h1>@Model.ListHeading</h1>
<p>
<a asp-action="Create">Create New</a>
</p>
<table class="table">
<thead>
<tr>
<th>
@Html.DisplayNameFor(model => model.BookList[0].ID)
</th>
<th>
… markup code removed for brevity
</th>
<th>
@Html.DisplayNameFor(model => model.BookList[0].DateUpdated)
</th>
<th></th>
</tr>
</thead>
<tbody>
@foreach (var item in Model.BookList) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.ID)
</td>
<td>
… markup code removed for brevity
</td>
<td>
@Html.DisplayFor(modelItem => item.DateUpdated)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { /* id=item.PrimaryKey */ }) |
@Html.ActionLink("Details", "Details", new { /* id=item.PrimaryKey */ }) |
@Html.ActionLink("Delete", "Delete", new { /* id=item.PrimaryKey */ })
</td>
</tr>
}
</tbody>
</table>
The Book View model is shown below:
Book View Model
using System;
using System.ComponentModel.DataAnnotations;
namespace BookLoanWebApp.Models
{
[Serializable]
public class BookViewModel
{
public int ID { get; set; }
[Required]
public string Title { get; set; }
[Required]
public string Author { get; set; }
public int YearPublished { get; set; }
public string Genre { get; set; }
public string Edition { get; set; }
public string ISBN { get; set; }
[Required]
public string Location { get; set; }
[Required]
public string MediaType { get; set; }
public DateTime DateCreated { get; set; }
public DateTime DateUpdated { get; set; }
public BookViewModel() { }
}
}
The Book List model is shown below:
Book List Model
using System.Collections.Generic;
namespace BookLoanWebApp.Models
{
public class BookListViewModel
{
public string ListHeading { get; set; }
public List<BookViewModel> BookList { get; set; }
public BookListViewModel()
{
BookList = new List<BookViewModel>();
}
}
}
One other thing to mention is that for hot reload to work for .NET Core MVC applications, you will need to install the following NuGet package into the application:
Enabling Hot Reload for an ASP.NET Core Application
One other thing to mention is that for hot reload to work for .NET Core MVC applications, you will need to install the following NuGet package into the application:
Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation
The Hot Reload feature is located on the toolbar of the development environment interface as shown to the right of the Continue button:

In the Hot Reload toolbar menu there is a menu item, Hot Reload on File Save. If we don’t check this item, then we will have to manually click on the Hot Reload menu item to force the modified code changes to be moved into the running application instance.
We can view the settings for Hot Reload by clicking on the Settings menu item:
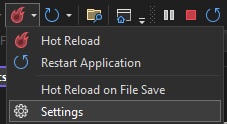
In the Options dialog within the Debugging | .NET / C++ Hot Reload sub-section we can see some options including enabling hot reload when not debugging and when code is changed during a breakpoint. The option to apply hot reload on code file saves is included.
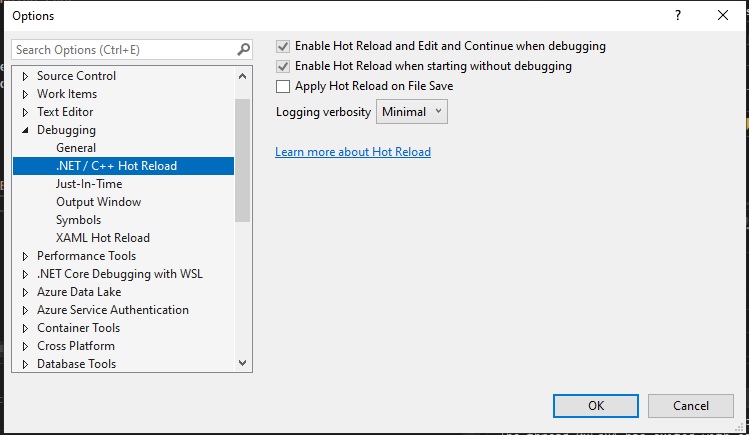
In the existing Startup.cs when you create an ASP.Net Core MVC project, you will see the following code to add views to the controllers:
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
}
To allow changes from CSHTML markup pages to be hot reloadable, add the extra services AddRazorPages() and AddRazorRuntimeCompilation() to the services collection as shown:
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
services.AddRazorPages().AddRazorRuntimeCompilation();
}
When the application is run, I will make the following changes:
- Add an additional ! character to the Welcome heading in the landing page.
- Add an additional ! character to the Book List heading in the book list page.
Below is the look of the book list view page when initially run:
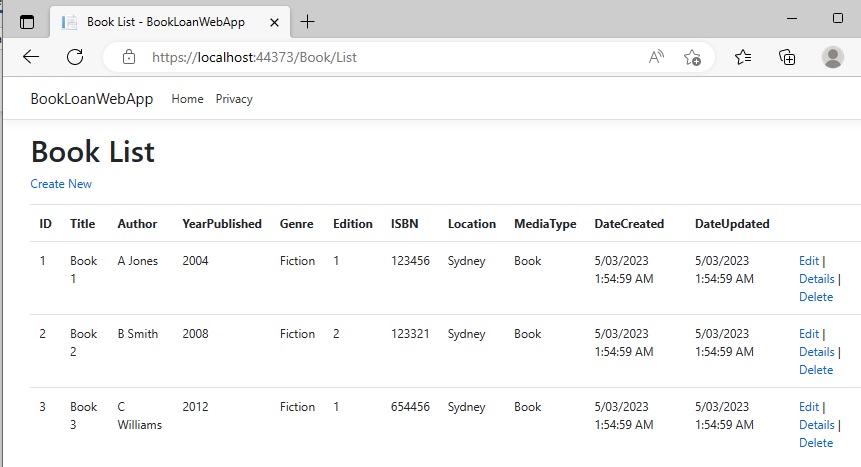
In the Index action method in the Home controller, I update the line of code as shown:
WelcomeMessage = "Welcome!!",
In the List action method in the Book controller, I update the line of code as shown:
ListHeading = "Book List!",
Now after refreshing the landing page and clicking on the View Books action under the Main Menu. There is no change. Why? Because I have not hit the Hot Reload action AND I have not checked the Hot Reload on File Save option.
I will now hit the Hot Reload action in the toolbar. You will notice in the status bar in the IDE showing the following message:

This means the code changes were applied to the running application instance.
If you were to see the following message, then it means that no code changes have been detected after actioning the last Hot Reload:

When we click on the Home link or refresh the landing page, we will see the heading updated with the modified value as shown:
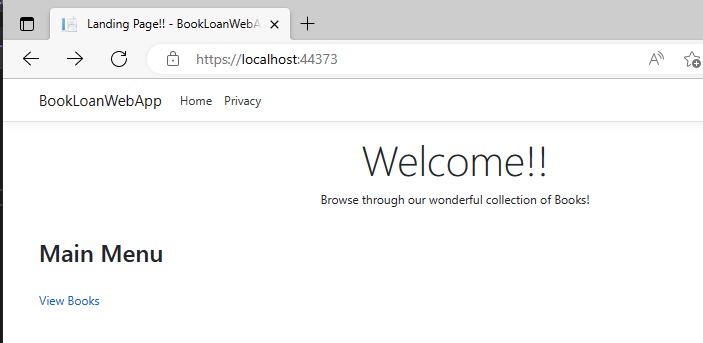
When we click on the View Books menu item in the landing page, the list heading will have changed as shown:
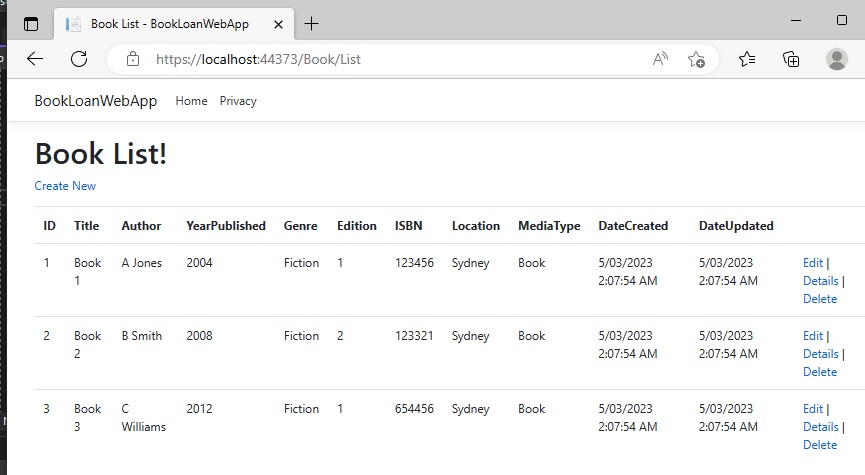
Next, I will show how the file save option works.
If we check the Hot Reload on File Save option as shown:
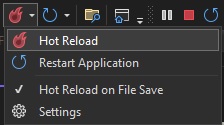
Then apply two additional changes as shown: In the Index action method in the Home controller,
I update the line of code as shown:
WelcomeMessage = "Welcome!!!",
In the List action method in the Book controller, I update the line of code as shown:
ListHeading = "Book List!!",
Now hit the Save All changes.
You will no longer see the message:

When we click on the Home link or refresh the landing page, we will see the heading updated with the modified value as shown:
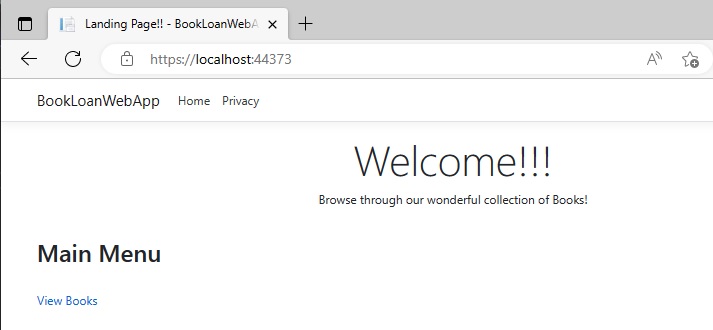
When we click on the View Books menu item in the landing page, the list heading will have changed as shown:
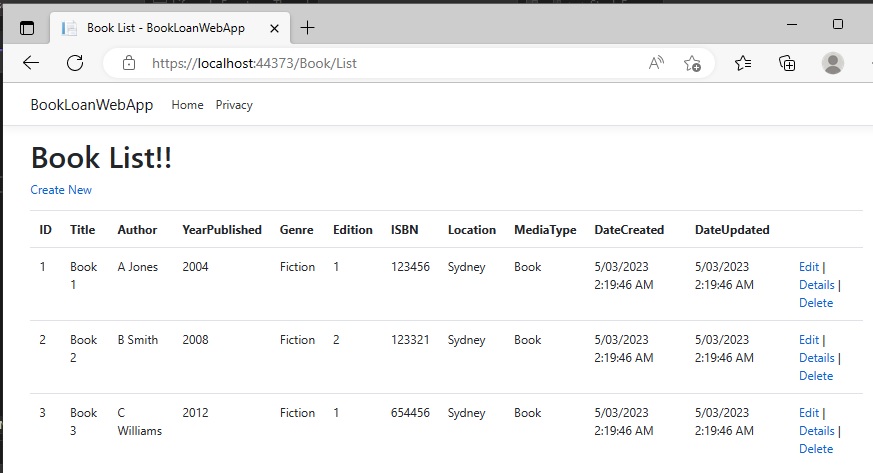
The above demonstrates Hot Reload on File Save works as expected.
To show how hot reload works for markup changes, uncheck the Hot Reload on File Save option, then open the Index.cshtml markup page and make the following change to the heading element:
<h2 style="color:blue">Main Menu</h2>
When the page is refreshed, there is no change, as expected with the markup showing no change in color:
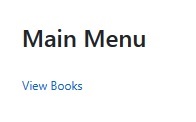
I will now hit the Hot Reload action in the toolbar. Then refresh the landing page. The markup will show a change in colour:
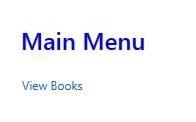
We will get a similar outcome when we check the Hot Reload on File Save option and apply a change to the markup in the Index page.
The above demonstration has shown us how to use the Hot Reload feature in Visual Studio for both manual code reloading and for code file saved reloads.
That is all for today’s post.
I hope you have found this post useful and informative.
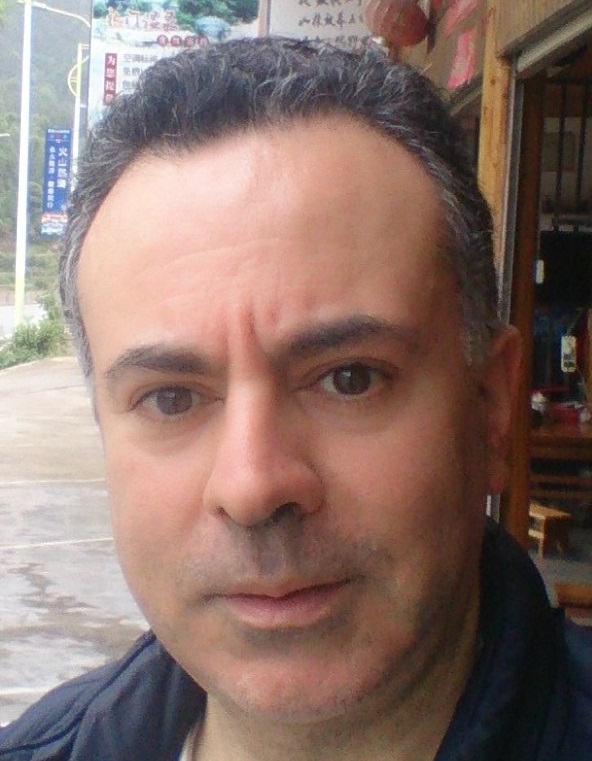
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.