Welcome to today’s post.
In today’s post I will look at how logging capability can be added to your .NET Core Web Applications.
I will be showing you how to use the logging middleware within .NET Core to add diagnostic logging output to the application’s debug console.
In another post, I showed how to output application logs to physical files in .NET Core. However, that approach requires use of a separate NuGet package library. In this post, the logging middleware is part of the .NET Core built-in libraries.
I will be showing how to inject the logging provider into startup class and into a typical controller class, from where we can send output messages to the log.
The examples I will be showing are done on as needed within the application, however as I showed in a previous post you can handle exceptions and include the log outputs within exception blocks, and even generate logs within a centralized handler within your .NET Core application.
Configuring Logging in the Application Startup
Logging can be configured is in Startup.cs and it is quite straightforward. To be able to use logging, the following namespace will need to be added in the using section of your source file:
using Microsoft.Extensions.Logging;
Next, the Configure() start up method will need the ILogger parameter as shown below:
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILogger<Startup> logger)
{
if (env.IsDevelopment())
{
..
}
else
{
..
}
..
To enable logging for different types of context use the logging service middleware to enable it within ConfigureServices() in startup.cs:
services.AddLogging(loggingBuilder => loggingBuilder
.AddConsole()
.AddDebug());
To call the logger and output some text, run a command like the one below:
logger.LogInformation("Application starting in development mode.");
When the application is run, the log output will be shown in the output window of your IDE as shown:
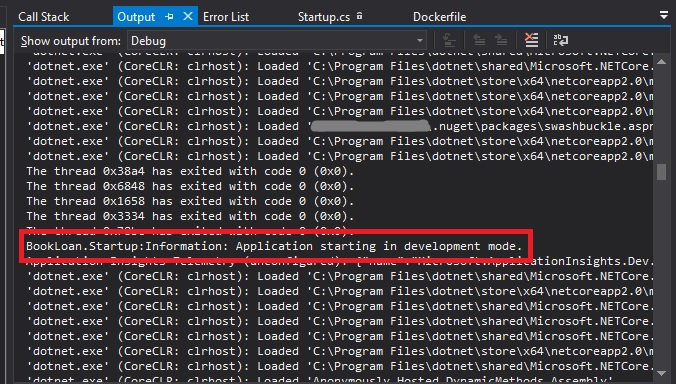
If your logging does not output to the debugger or standard console then check your appSettings.json for correctly configured logging files:
{
"Logging": {
"IncludeScopes": false,
"Debug": {
"LogLevel": {
"Default": "Information"
}
},
"Console": {
"LogLevel": {
"Default": "Information"
}
}
}
}
If you specify the default log level to “Warning” or “Error” and output an information message, then the output will not show as it does not match the filter.
Configuring Logging within a Class
To configure services or custom classes or even controllers to use logging, you can use constructor injection as shown:
ILogger _logger;
IBookService _bookService;
public SearchController(ApplicationDbContext db, IBookService bookService, ILogger<BookController> logger)
{
_db = db;
_logger = logger;
_bookService = bookService;
}
Note: If you attempt to use the ILogger constructor parameter as shown:
ILogger logger
Then you will end up with the following error:
An unhandled exception occurred while processing the request.
InvalidOperationException: Unable to resolve service for type 'Microsoft.Extensions.Logging.ILogger' while attempting to activate 'BookLoan.Controllers.SearchController'.
Microsoft.Extensions.Internal.ActivatorUtilities.GetService(IServiceProvider sp, Type type, Type requiredBy, bool isDefaultParameterRequired)
The reason for this is that each ILogger instance needs to be created with an instance of a type T, which is a category type.
The category type for constructor injection corresponds to the container class.
During debugging of the ILogger instance, you can see the category type corresponds to the type of the class T that the logger is injected into as shown:
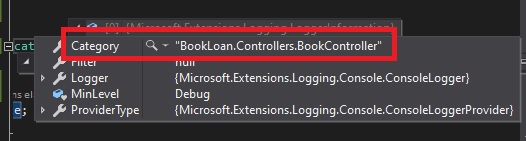
When output, the log will show the fully qualified category type name as shown and the output message:
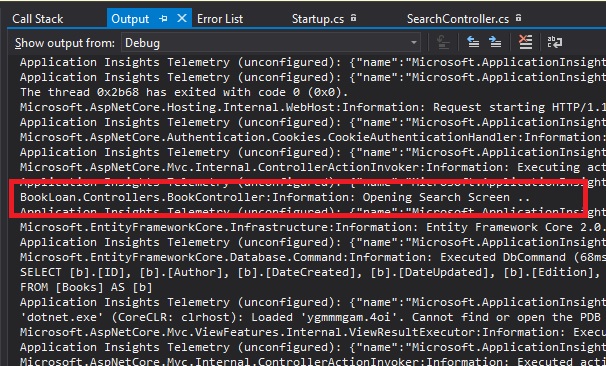
For more details refer to the .NET Core documentation on logging.
We have seen how to configure logging within a .NET Core application and seen how it is configured within the following areas of the application:
- Application startup.
- Classes.
- Controllers.
We have also seen how to set the filtering of the logs to control the verbosity Warning, Error, Info etc. of logs by using the Logging key in app settings.
We also saw how to read the debug console for log output.
I also showed how to correctly declare the logging service as a category type that matches the type of the class that we are logging.
That’s all for today’s post.
I hope you found it informative and useful.
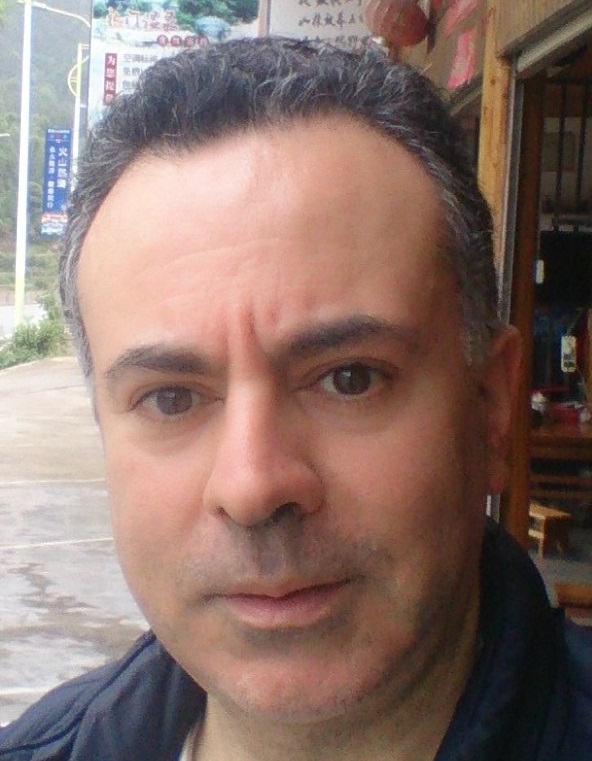
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.