Hi and welcome back to my blogs on development!
In today’s blog I will go over what application settings are and how they are used within .NET Core applications.
I will also explain how you can apply application settings within your .NET Core applications using some useful dependency injection patterns built into .NET Core 2.x.
In the first section, I will explain what application settings are in a .NET Core application.
Settings Storage in .NET Core Applications
Every application that is built with .NET Core reads configurations from a separate settings file. The settings file is physically stored in the same folder as the application project file.
The file used to store configuration settings for our .NET Core web application is the appsettings.json file. The structure of settings keys and values is in the JSON data format. A sample format of this file is shown as follows:
{
"ConnectionStrings": {
"AppDbContext": "[some connection string]"
},
"Logging": {
"IncludeScopes": false,
"LogLevel": {
"Default": "Warning"
}
},
"AppSettings": {
"AppName": "BookLoan",
"AppVersion": "1.0.0",
"AlertEmail": "operator@bookloan.com"
}
}
What you can see is there are different sections in the configuration file. In the above file we can see a section, ConnectionStrings for storing connection strings, a section, Logging to store configurations for application logging diagnostics, and a section, AppSettings for storing general application configuration settings.
To obtain a specific value from a connection string within the configuration settings file, we can use the following command:
string dbConnStr = Configuration.GetConnectionString("AppDbContext")
The Configuration instance is injected through a constructor and the IConfiguration interface, from Startup():
public IConfiguration Configuration { get; }
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
This is quite straightforward, and like what we see with traditional .NET 4.x Framework applications.
Next, I will explain how configuration values can be read from the AppSettings section.
Reading Configuration Values from AppSettings
As we do for connection strings, access is through the Configuration instance. The configuration instance has a useful method, GetValue<Type>() that allows retrieval of configuration values of most scalar types (integer, string, integer).
Configuration values are specified in the following format:
[Section]:[Configuration Key]
Below is a code sample that reads in the application name and application version from the configuration keys within the AppSettings section:
string sAppName = Configuration.GetValue<string>("AppSettings:AppName");
string sAppVersion = Configuration.GetValue<string>("AppSettings:AppVersion");
If we have many settings and key/value pairs to retrieve into our service classes, the above would be quite cumbersome and would require repeating for each value within a configuration section. The solution to this is to strongly type the app settings section into a class and take advantage of dependency injection built into .NET Core.
These are the steps we can take to achieve this:
Step 1 – Create a class to contain your application settings
In the ConfigureServices() method within startup.cs add the following two lines:
using System;
namespace BookLoan.Services
{
public class AppConfiguration
{
public AppConfiguration() { }
public string AppName { get; set; }
public string AppVersion { get; set; }
}
}
Step 2 – Update ConfigureServices()
In your ConfigureServices() method within startup.cs add the following two lines:
public void ConfigureServices(IServiceCollection services)
{
… services.Configure<AppConfiguration>(Configuration.GetSection("AppSettings"));
…
}
Include also the Microsoft.Extensions.Configuration namespace at the top of the source.
To bind property values within the AppConfiguration instance to the corresponding configuration keys within the AppSettings section within appsettings.json, we used the following line of code:
services.Configure<AppConfiguration>(Configuration.GetSection(“AppSettings”));
The following command:
services.Configure<AppConfiguration>(Configuration.GetSection("AppSettings"));
allows your options to be bound to the respective configuration key from appsettings.json.
[See also here for more a detailed reference.]
Step 3 – Access the settings from your class
To be able to set the application configuration within a service class within the application, we need to first reference an injected instance of the configuration class in a service class constructor. configuration values, which are properties within the configuration instance are accessed through other methods within the service class.
namespace BookLoan.Data
{
public class SeedAccounts
{
private ApplicationDbContext db;
private AppConfiguration appConfiguration;
public SeedAccounts(ApplicationDbContext _db, AppConfiguration _appConfiguration)
{
db = _db;
appConfiguration = _appConfiguration;
}
public async Task ConfigureApplicationFeatures()
{
string appversion = appConfiguration.ApplicationVersion;
..
}
..
}
In this post, we have learnt the following about configurations within a .NET Core application:
- How application configurations are setup within a .NET Core application appSettings.json file.
- How configurations are read in from an application using dependency injection.
- How properties within instances of a class can be bound to application settings.
The application settings that we have stored within the appSettings.json file isn’t only used for configuring the application in an on-premises web server. It can also be used for settings within the same web application when it is deployed into an App Service instance in Azure. In a future post I will explain how the same settings are used in the context of a web application hosted in an Azure App Service plan instance.
That is all for today’s post.
I hope you have enjoyed this post and find it useful.
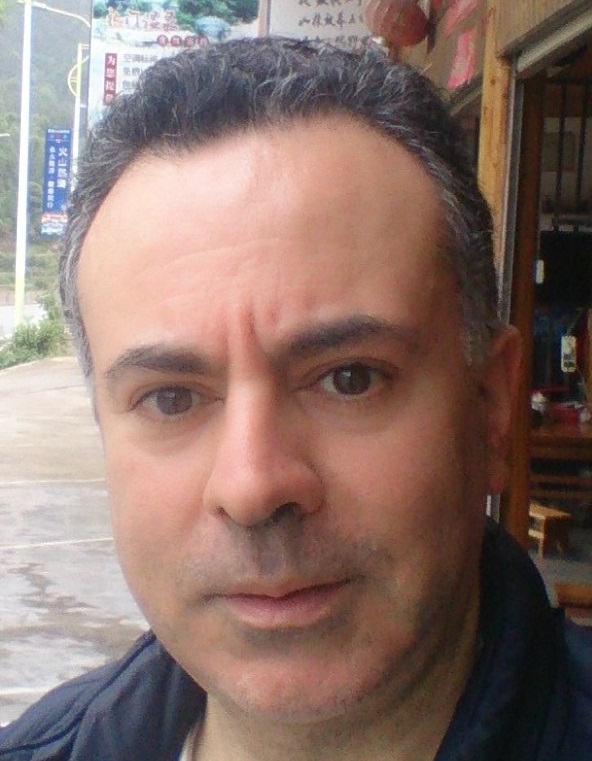
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.