Welcome to today’s post.
In today’s post I will be discussing how to use TSLint to improve your TypeScript and JavaScript source code quality within an Angular project.
In a previous post I explained how to improve code quality with refactoring. In today’s post I will show how to improve the quality of your source code readability and how to standardize the syntax according to the currently used development language and libraries.
In this post I will explain why as developers, we should be using linting tools. I will then show how TSLint is setup within an Angular application. I will then conclude by showing some examples of how to use TSLint in the Visual Studio Code development environment.
Why use Linting Tools During Development?
Without the use of a linting tool, there is a risk that the developers working on the same code base will not be able to apply uniform code quality rules or improve on the quality of the code base.
As developers we are human and more often, remembering static compiler rules on syntax and compiler conventions is not one of our biggest strengths. As a code base changes during a project lifetime, and development libraries are upgraded within a project, rules on syntax will be ever changing, and as developers, being able to keep track of changing syntax rules is something that developers do not need to be burdened with. Moreover, the most common readability rules, such as ensuring indentation of if..then..else code blocks and ensuring spacing when required are unchanged irrespective of the language or library versions we use.
Given these considerations, the use of linting tools is encouraged to ensure common uniform standards in implementing code readability are practiced within a development team and not allow the bad habits to accumulate with time. As with any code base, neglecting the code readability is also a genuine technical debt and accumulates over time.
The TSLint Tool in Angular
With any Angular project you create (using ng new), the default linting tool, TSLint is included with the package of extensions loaded with the scaffolded application. This is shown with the creation of the tslint.json configuration file in the application source folder.
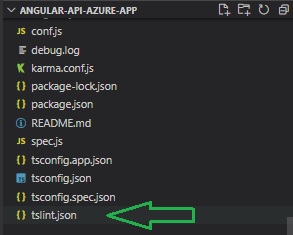
In the package.json file, the dev dependencies will include the tslint NPM package version as shown:
"devDependencies": {
"@angular-devkit/build-angular": "~0.1002.3",
...
"tslint": "~6.1.0",
"typescript": "~4.0.8"
}
The basic scripts used to build, start, test and lint the project will look as shown:
"scripts": {
"ng": "ng",
"start": "ng serve",
"build": "ng build",
"test": "ng test",
...
"lint": "ng lint",
"e2e": "ng e2e"
},
The Angular.json file will also have default configuration to lint TypeScript code as shown:
"lint": {
"builder": "@angular-devkit/build-angular:tslint",
"options": {
"tsConfig": [
"tsconfig.app.json",
"tsconfig.spec.json",
"e2e/tsconfig.json"
],
"exclude": [
"**/node_modules/**"
]
}
},
In the above, the node,js modules are excluded from the linting processor.
The tslint.json file specifies many linting rules. Among these are the following rule:
"quotemark": [
true,
"single"
],
Running the linting tool is quite straightforward. Use the command:
ng lint
Depending on the size of your code base and the quality of your code, you will see output that contains quality analysis in the following columns:
Quality Type: ERROR or WARNING
Affected Line and Column
Linting rule
Description of quality issue
Below is sample output showing the errors and code syntax correction recommendations:
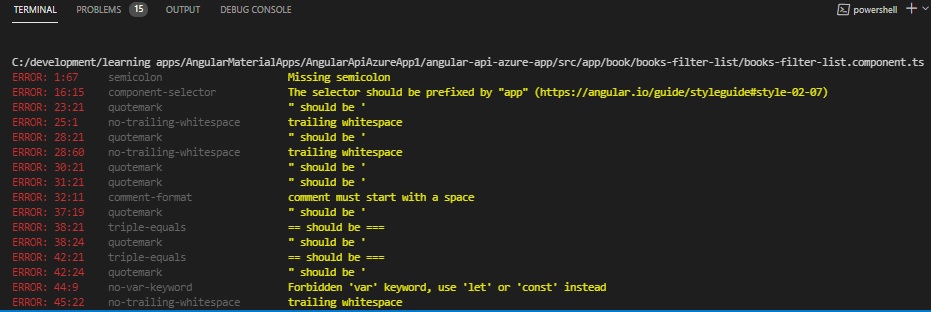
In the next section, I will go over some examples on how TSLint is used within the Visual Code development environment.
Examples in usage of the TSLint Tool
In this section I will now pick out some sample linting issues that we can encounter during development within the Visual Studio Code environment and indicate the affected source code.
Below is the linting analysis for the quotation rule, equality operator === and appropriate use of the var declaration:
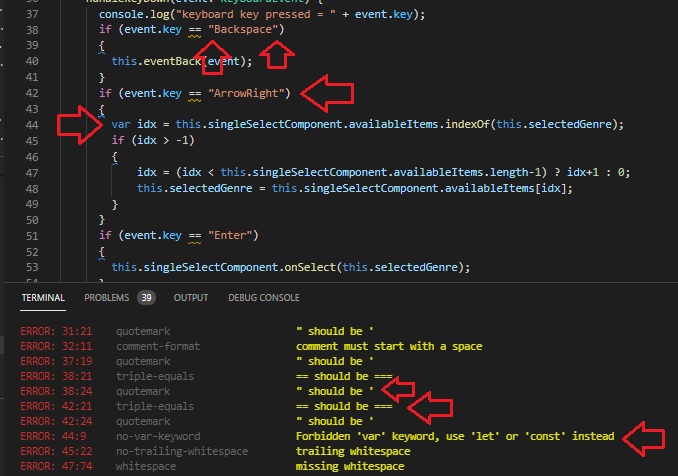
As we can see, if you are a full-stack developer that frequently uses a backend compiled language such as C#, the use of the double quote character to specify strings is quite common and when switching back to TypeScript/JavaScript we persist with the habit on our front-end scripting.
In the next example, the linting tool can find very difficult to find quality issue of trailing whitespace:
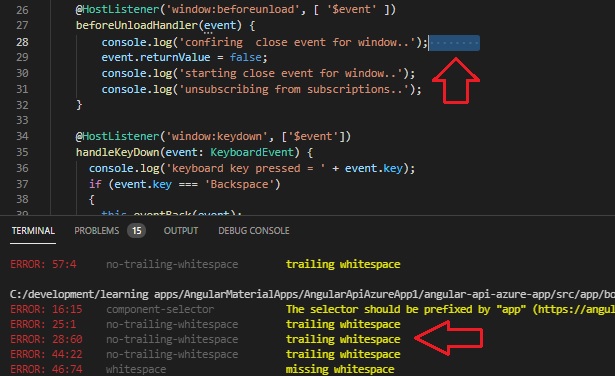
Even though the code will in nearly all cases be able to build and run without issues, there are some situations where white space can be the cause of some extremely difficult to find bugs.
An additional readability issue is the lack of missing whitespace as shown below:
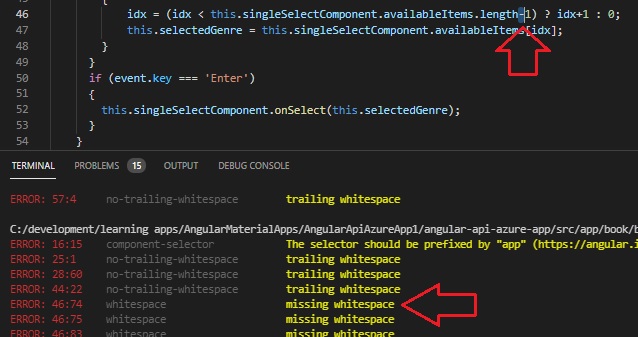
The use of whitespace makes our code more readable without impacting the quality.
Another example is the lack of opening and closing braces within a conditional statement and misaligned lines of code. These are shown below:
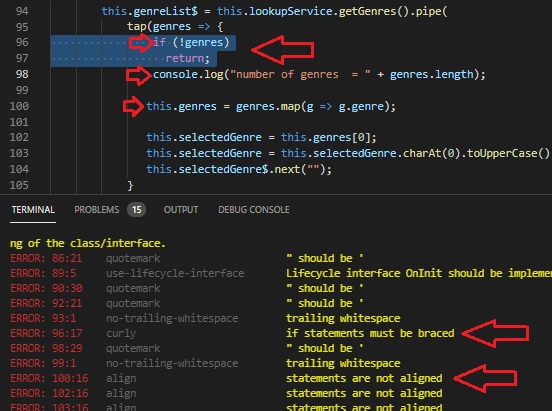
The lack of braces can make complex conditional statements less ambiguous by enclosing conditional blocks within braces. Aligning code correctly makes it easier for coders and reviewers to associate code that is within the same scope block.
In the final example we see a library syntax issue flagged that shows the version of the functional prototype used is deprecated:
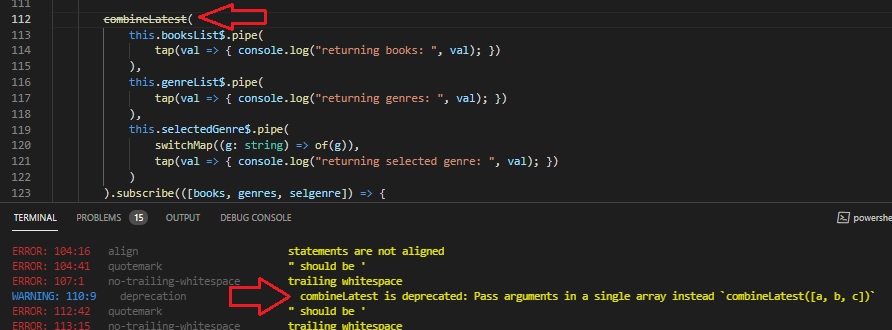
In this case, the version of the combineLatest() method from the RxJS library does not use the latest version of the prototype and parametrization to enclose the parameters within an array.
As we can see, by applying some of the most common and basic syntactic and readability rules while we are developing, we can improve the quality and readability of our code base and make it more readable for other developers after check-in to our code repository.
From late 2019, the use of TSLint was deprecated in favour of an upgraded linting tool, ESLint. for new projects using Node,js 12.2 and above and Angular 11, the ESLint extension is included by default. For existing projects prior to Angular 11, the TSLint extension can continue to be used.
In a future post I will show how to use ESLint and how to upgrade from TSLint within an Angular application.
That is all for todays post.
I hope you have found this post useful and informative.
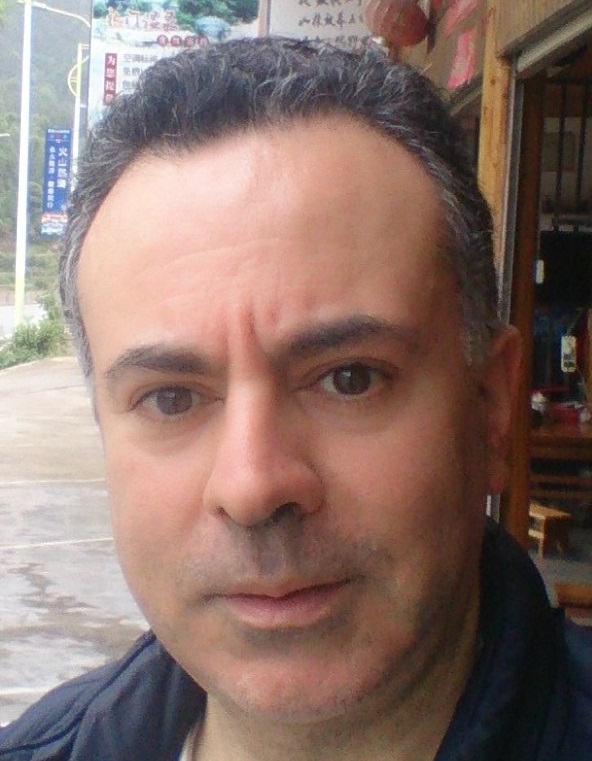
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.