Welcome to today’s post.
In today’s post I will discuss the various ways we can make use of TypeScript within an Angular application to check values and objects against null and undefined values.
The various operators discussed in this post are already used quite extensively within JavaScript, as TypeScript is essentially an extension of JavaScript EcmaScript 6 (ES6) with many features thrown in.
So, for those developers who are new to TypeScript but not so familiar with JavaScript, they will provide a useful set of programmatic idioms for comparing values against null and undefined values. Given their similarities, the comparison statements can be usefully applied for applications written with either of the two scripting languages. By applying the comparison idioms consistently, we can make our code more compact and readable for object comparisons.
I will start by showing how we can check on the following combinations of null, not null, and undefined objects with Typescript:
Not-null objects and values
Null objects and values
Undefined objects and values
We will start first with checking for not-null objects and values in the next section.
Checking for not null objects and values
Consider the situations where we use a condition to check for a not-null object or value.
In this case, suppose that we want to check the truth of a value, my_value.
We use the following statement:
if (my_value !== null)
{
…
}
We could also use the form:
if (my_value)
{
…
}
The above shows the use of the truthy. According to the MDN reference of the definition of Truthy, the truthy value from a JavaScript definition is a value that is considered as a true value.
Typical examples of truthy values are:
true
1
“1”
{}
[]
The opposite is a falsy, which is a value that is considered as a false value.
Typical examples of falsy values are:
false
null
0
“”
Checking for null objects and values
Consider the situations where we use a condition to check for a null object or value.
In this case, suppose that we want to check the nullity (or falseness) of a value, my_value.
We use the following statement:
if (my_value === null)
{
…
}
We could also use the form:
if (!my_value)
{
…
}
The above forms check for the falsy of a value.
Checking arrays for truthy or falsy
Unlike basic value types, arrays are slightly more complex to test for truth or false conditions. Arrays comprise of more than one object or value.
The above checks we used on values for truthy or falsy testing do not apply for arrays:
Consider the following condition:
if (!x)
The above condition will not test for an empty array.
To check for an empty array, we use:
if (myArray.length === 0)
Use of optional chaining to short-circuit null property values
In the above case when we checked an array for nullity, we forgot to cover one other case. What if the array, myArray is undefined or null? In this case, we can optimise the condition on an array, object instance, or structure by short-circuiting the condition.
This is where we use the optional chaining operator (?) to short-circuit evaluation of a null object. The optional chaining operator is the question mark (also known as the Elvis operator).
A detailed definition of optional chaining is in the following reference link.
In the above array, we can use short-circuiting to check the length of an array as follows:
if (myArray?.length === 0)
If we have objects defined like this:
objectA: any = {
a: string;
}
And this:
objectB: any = {
b: string;
x: objectA;
}
To check for property a within objectA via objectB we try:
objectB.x.a
With safe navigation we use:
objectB?.x?.a
The above ensures that if objectB is null or undefined, or the property x within objectB is null or undefined then the expression evaluates without error. Essentially, we save having to use a nested if … else condition or a condition with multiple Boolean && operators like this:
if ((objectB !== null) && (objectB !== undefined) && objectB.x && objectB.x.a)
or like this:
if ((objectB !== null) && (objectB !== undefined))
{
if (objectB.x && objectB.x.a)
{
…
}
}
Using the exclamation operator to assert a value is not null
When strict type checking is enforced, we can ensure that a value is not null and not undefined by following it with the exclamation (!), (or bang) operator. So below, we can ensure the value x is not null and not undefined by using:
x!
During runtime, when the value x is null or undefined, we will get an error saying that x is undefined. The above however, does not prevent x from being null or undefined.
Refer to the typescript language reference link to explain the exclamation operator.
Using the null-coalescing operator to check for nullity
Like we did to conditionally check object properties for validity, we can apply a similar check within an expression to provide defaults for a null or undefined value.
The expression below:
let x = objectB?.x?.a ?? “Default Value”
will evaluate to “Default Value” if the property value objectB?.x?.a is null or undefined.
It replaces the following if .. else condition:
if (objectB?.x?.a)
{
x = objectB?.x?.a;
}
else
{
x = “Default Value”;
}
As we have seen, there are some useful shortcuts in TypeScript (and JavaScript) that we can utilize to evaluate objects and values within an application. This allows us to keep our code cleaner, more readable and testable. Many of the above constructs are identical to those found in the syntax found in the c, c++ and c# programming languages, so if you are familiar with any of those languages, the constructs used in TypeScript and JavaScript will be familiar.
That is all for today’s post.
I hope you have found this post useful and informative.
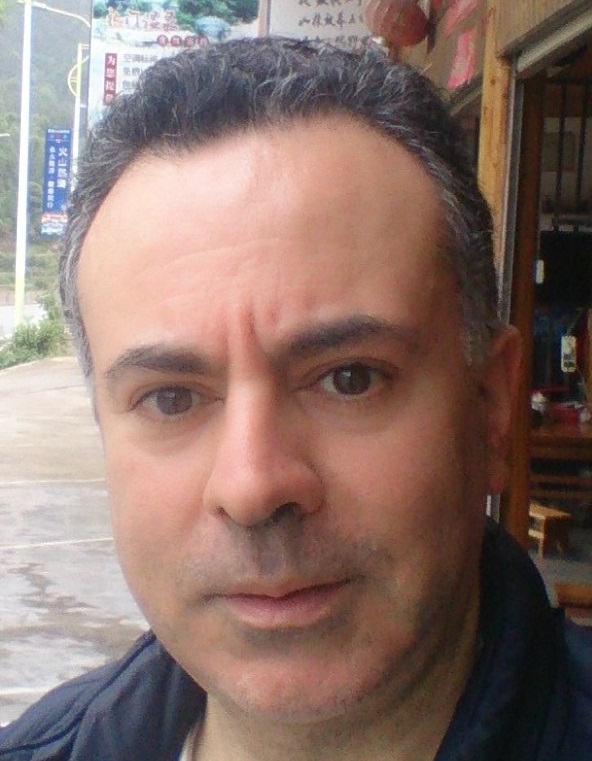
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.