Welcome to today’s post.
In today’s post I will show how to set a default input date with the Angular Material Date Picker component.
In a previous post, I showed how to use the Material Date Picker component within an Angular application. I explained how to set the input date using a JavaScript date object which is set from an external Web API service.
In today’s post I will expand on that discussion by showing how to assign the input date correctly and compare incorrect and correct date formats and how they affect the date picker input control and drop-down display to the end user.
I will show how this is done with an Angular Reactive form control.
Material Date Picker within an Angular Reactive Form Control
Below is the standard Angular Reactive form template for a Material Date picker control:
<mat-card-content>
<form [formGroup]="meetingForm">
<div class="form-group">
<label for="meetingDate">Meeting Date:</label>
<br />
<input
matInput
[matDatepicker]="meetingDate"
formControlName="meetingDate"
(dateInput)="addEvent('input', $event)"
(dateChange)="addEvent('change', $event)" />
<mat-datepicker-toggle
matSuffix
[for]="meetingDate">
</mat-datepicker-toggle>
<mat-datepicker #meetingDate startAt="meetingDate">
</mat-datepicker>
</div>
</form>
</mat-card-content>
Setting the default input date to the data picker can be done within the ngOnInit() event, which I will show later.
Setting the default date requires the input date to be an ISO compliant date string.
An ISO compliant date string is of the format:
YYYY-MM-DD
We can achieve this with at least two different methods:
- Create a JavaScript Date object and set the constituent date parts.
- Use date string valid for the browser local then convert it to an ISO date string.
Using a JavaScript Date object
Below is an example of using a JavaScript Date object, which uses an ISO date format.
Suppose we have a date: 24/8/2021 that we would like to set as the default date. After creating the current date object, we assign the constituent date parts as shown:
private initForm(fb: FormBuilder) {
let currDate = new Date();
currDate.setDate(24);
currDate.setMonth(8);
currDate.setFullYear(2021);
...
We then convert the date string to ISO format as shown:
let currDateString: string =
currDate.getFullYear().toString() +
'-' +
this.padStart(currDate.getMonth(), 2) +
'-' +
this.padStart(currDate.getDate(), 2);
Where padStart() is a user-defined function like the one shown:
padStart(num, size) {
num = num.toString();
while (num.length < size) num = '0' + num;
return num;
}
Or if you are using ECMA 2017, then padStart() is a built-in function, so the above ISO date string calculation can be simplified to:
let currDateString: string = currDate.getFullYear().toString() + '-' +
currDate.getMonth().toString().padStart(2, '0') + '-' +
currDate.getDate().toString().padStart(2, '0');
In the next section, I will show another way to retrieve the current date as an ISO compliant date.
Using the ISOString() function
Finally, using the toISOString() function for JavaScript dates can be used to extract the ISO date string from our date object as shown:
let currDateString: string = currDate.toISOString();
A reference to the toISOString() function is located in the JavaScript reference on the Mozilla developer site.
The converted date is then set as a default within the date picker form control within the form control group as shown:
this.meetingForm = fb.group({
meetingDate: [currDateString, Validators.required],
});
}
When the application is refreshed, the input date will then be shown correctly as shown:
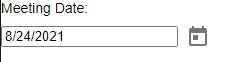
The input selectable date highlighted in the date picker drop down is shown below:
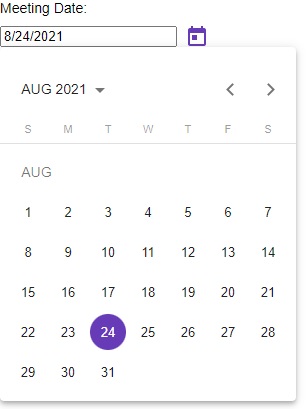
The above date as we have seen work correctly with the ISO format:
let currDateString: string = '2021-08-24';
Had we attempted to simply set the input dates as follows:
let currDateString: string = '24/08/2021';
OR
let currDateString: string = '2021-24-08';
The date picker would show the input date as a blank as shown:
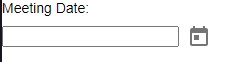
The date picker has not accepted a date in any other locale format such as DD/MM/YYYY or MM/DD/YYYY.
When the input date is not accepted as valid, the date picker will display the default date as the current date as shown:
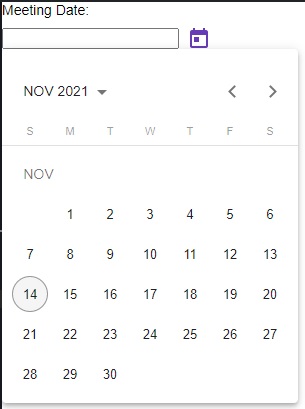
To summarize, in our component source we initiate the current date value for the form controls used in the Material Date Picker as follows.
Through the component constructor we do this as shown:
constructor(private router: Router, private fb: FormBuilder) {
this.initForm(fb);
}
private initForm(fb: FormBuilder) {
let currDate = new Date();
const currMonthNum = currDate.getMonth() + 1;
let currDateString: string = currDate.getFullYear().toString() + '-' +
currMonthNum.toString().padStart(2, '0') + '-' +
currDate.getDate().toString().padStart(2, '0');
this.meetingForm = fb.group({
…
meetingDate: [currDateString, Validators.required],
…
});
}
In the NgOnInit() lifecycle event, we can se the default date in the Date Picker as shown:
ngOnInit(): void {
console.log("MeetingNew Component instantiated");
let currDate = new Date();
…
const currMonthNum = currDate.getMonth() + 1;
…
let currDateString: string =
currDate.getFullYear().toString() + '-' +
currMonthNum.toString().padStart(2, '0') + '-' +
currDate.getDate().toString().padStart(2, '0');
…
this.meetingForm.controls['meetingDate'].setValue(currDateString);
…
}
As we have seen from the above example, when setting input dates in the Material Date picker, the use of current dates that are locale dependent gives dates in incompatible date formats. We avoided this issue by using one of two methods to convert the default input date into a YYYY-MM-DD formatted string, which is ISO format compliant.
We did this by converting the date, which was instantiated as a JavaScript object into an ISO format date using either by building it by using the JavaScript string function padStart(), ormake use of the toISOString() function to convert our current date into a date format that is compatible with the Material Date Picker.
That is all for today’s post.
I hope you have found this post useful and informative.
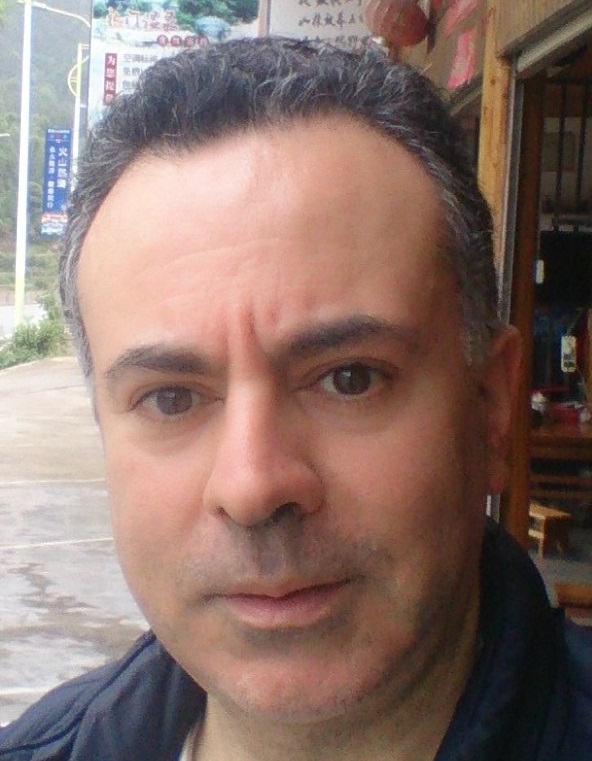
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.