Welcome to today’s post.
In todays post I will be explaining how to test gRPC .NET Core services using a variety of tools.
In previous posts I showed how to implement a gRPC .NET console client which allowed us to test connectivity and execute calls using different RPC call types to a gRPC service library.
If we had just implemented a gRPC service library and wished to setup and run some tests without having to implement a client tester application, then the option of using a tool to conduct the testing of requests and responses would also useful.
One of the tools I am suggesting is the Postman API testing tool.
In previous posts I have showed how to use Postman to write test scripts that can be run tests for .NET Core web API requests and responses manually and run test scripts from Postman automatically.
In the first section, I will first show how to setup Postman to test gRPC service calls.
Setup of Postman for Testing of Calls to gRPC Services
In the earlier versions of Postman there were no features that allowed for the testing of gRPC services, however since version 10, the capability to be able to test the execution of requests and responses to a gRPC service is available. In addition, the test interface for gRPC services allows for the testing of the four different call types: unary (one-way), client stream, server stream, and duplex (bidirectional).
With earlier editions of Postman, the Scratchpad allowed for the storing of collections of requests. Since the release of version 10 of Postman, the Scratchpad has been replaced with an online equivalent called the Workspace that allows for the saving of collections of requests. In addition, the provision of gRPC testing is only available in the Postman online Workspace.
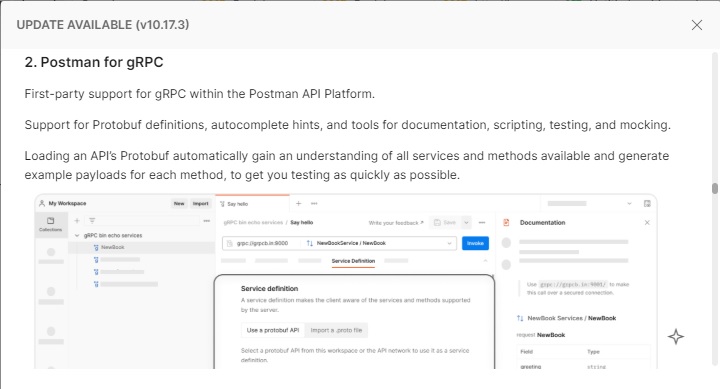
I will now show how to setup gRPC test calls within the Postman Workspace.
To create a gRPC test request select the New request option, then in the next screen select the gRPC option as shown:
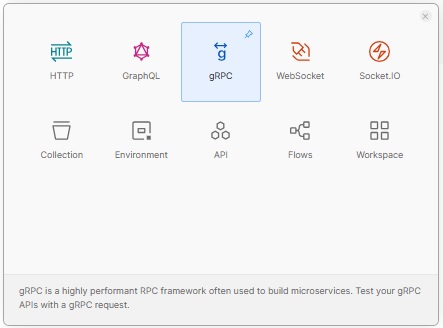
You will now see an untitled request. This requires a URL and a method to be selected.
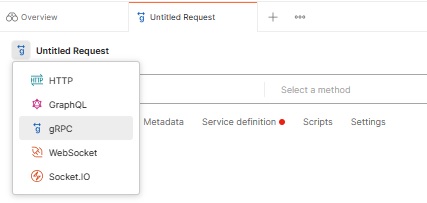
If we are not aware of the URL endpoint of the gRPC service, we can refer to the configuration of the gRPC service or run the service and inspect the debug console for the displayed (http or https) URLs.
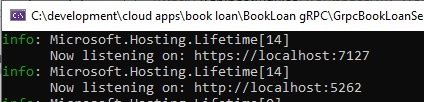
With the gRPC service I am running locally, the port is 5262 (which may differ in your setup), so the URL I enter in the URL request is:
http://localhost:5262
Next, we select the method edit. A drop down will show. You may initially not see any methods show in the drop-down list.
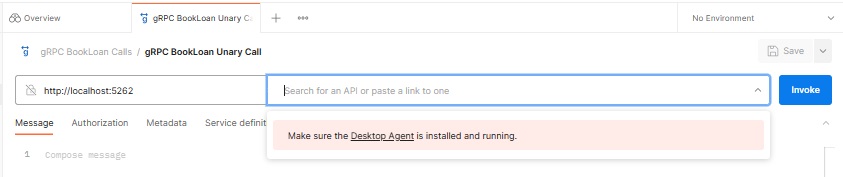
To see the methods for the gRPC service we will need to have the following requirements:
- The gRPC service must be running.
- The machine you are running Postman will need to have Postman Desktop Agent running.
- The gRPC server will need to either support reflection and discovery of the service contract methods OR Postman will need to have the Protocol buffer (Protobuf) file for the gRPC services imported.
If the link in the method drop-down list is selected, you will see the following prompt:
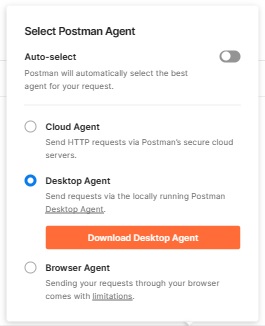
Select the Desktop Agent to download.
After downloading the desktop agent and installing it, re-select the drop-down list. You should now see three additional options available:
- Use a Protobuf API
- Import a Protobuf file
- Use Server Reflection
Select the option Use Server Reflection.
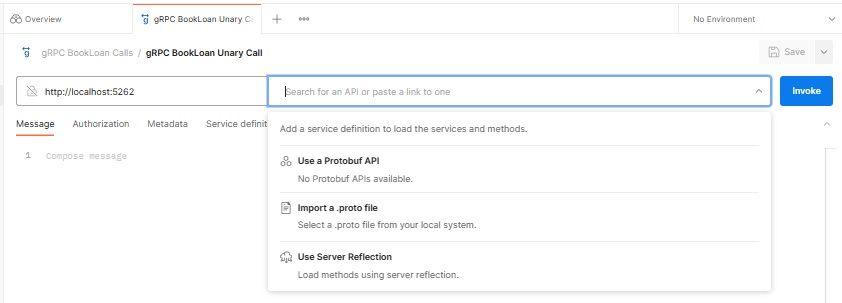
If your gRPC .NET Core service is currently running and you notice the following error:
Could not load server reflection
The above error is shown in the drop-down list for the API service loading:
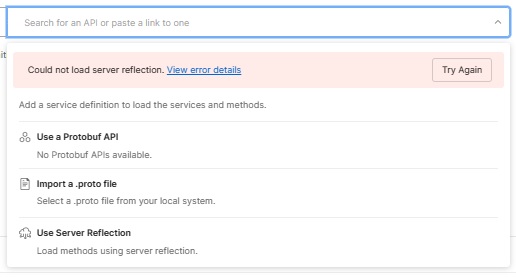
Or you could see the following error:
UNIMPLEMENTED: Service is unimplemented
The same error shows in the Service definition tab as shown:
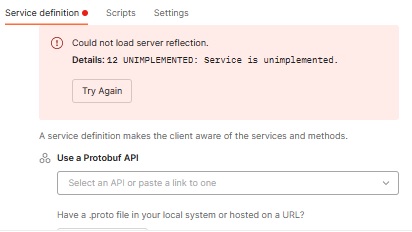
And then see a similar error in the debug console of the running gRPC server in Visual Studio:
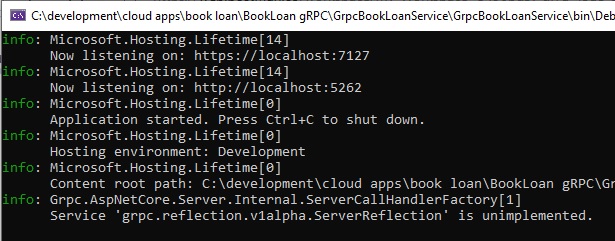
Then you will need to make a few small changes to the gRPC service, re-build it, and run it again before re-attempting the RPC request from Postman.
The first error is likely to be caused by the gRPC service library not having the Protobuf service contract definitions not visible by server reflection.
After opening the gRPC Server .NET Core project in Visual Studio 2022, open the NuGet Package Manager and install the package:
gRpc.AspNetCore.Server.Reflection
Which is shown in the NuGet Package Manager as shown:
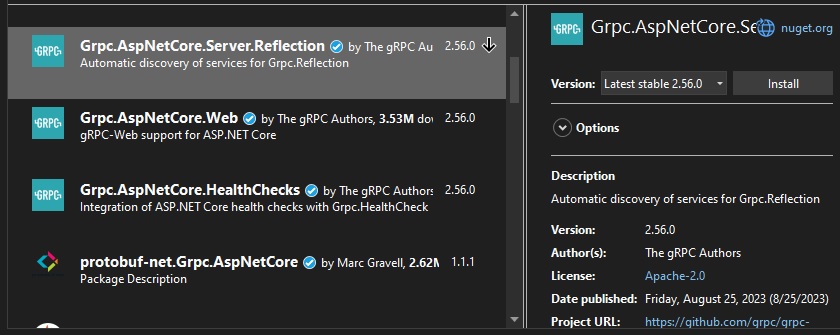
After installation, you should have both gRPC.AspNetCore.Web and gRpc.AspNetCore.Server.Reflection packages installed into the project:
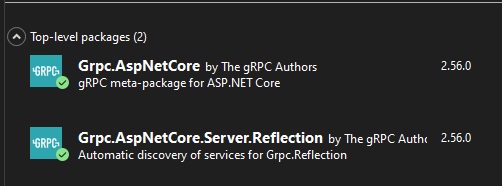
In the Program.cs startup file you will need to include the following line of code to add the server reflection to the gRPC service’s server collections:
builder.Services.AddGrpcReflection();
In addition, to avoid the second UNIMPLEMENTED service error, you will need to include the following line of code to the application pipeline:
app.MapGrpcReflectionService();
The code to bootstrap the gRPC service is shown below:
using GrpcBookLoanService.Services;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddGrpc();
builder.Services.AddGrpcReflection();
var app = builder.Build();
// Configure the HTTP request pipeline.
app.MapGrpcService<LibraryService>();
app.MapGet("/", () => "Communication with gRPC endpoints must be made through a gRPC client. To learn how to create a client, visit: https://go.microsoft.com/fwlink/?linkid=2086909");
IWebHostEnvironment env = app.Environment;
if (env.IsDevelopment())
{
app.MapGrpcReflectionService();
}
app.Run();
Now build and run the gRPC service.
In the Postman gRPC request in the Service definition tab, the Using server reflection will show with a tick to indicate the server reflection is running within the service:
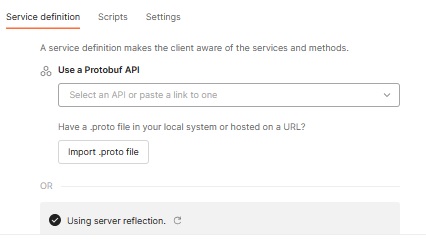
After selecting the server reflection option, the service methods will be visible and listed in the drop-down list:
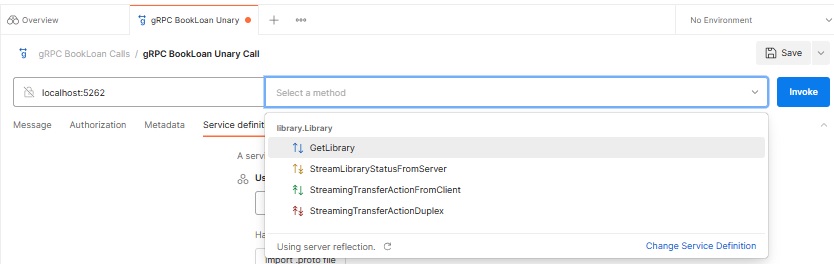
In the next section, I will show how to test RPC Unary calls.
Testing an RPC Unary (One-Way) Call
I will now show how to test a basic unary RPC call.
I will select the unary call, GetLibrary.
I then select the Message tab and enter the parameter:
{
“library_id”: 1
}
Now click the Invoke button to execute the RPC call.
The result (if successful) will show in the response tab:
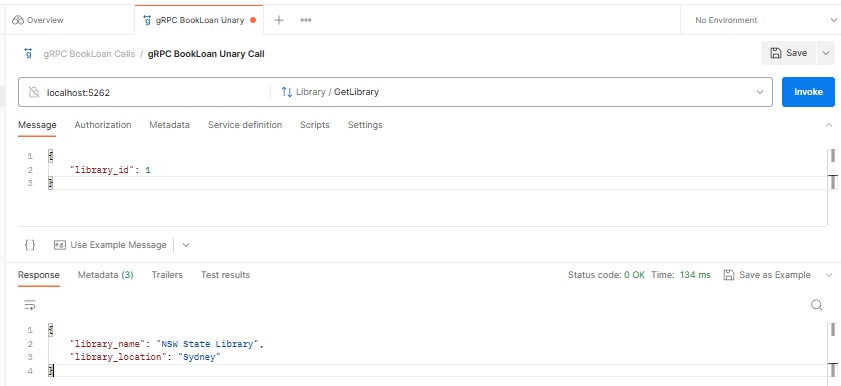
We have just successfully tested an RPC unary server call to a gRPC service within Postman.
If the gRPC service was not running when we ran the RPC request from Postman, then the following error would show in the method drop-down list:
UNAVAILABLE: No connection established
In this case, the server reflection from the gRPC service would not load. The above error is displayed within the Service definition tab as shown:
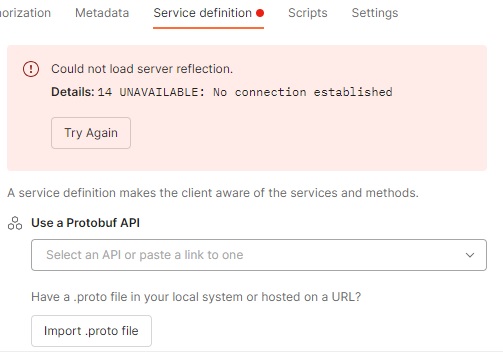
In the next section, I will show how RPC Server Stream calls as tested.
Testing an RPC Server Stream Call
I will now show how to test a server stream RPC call.
I will select the server stream RPC call, StreamLibraryStatusFromServer.
I then select the Message tab and enter the parameter:
{
“library_id”: 1
}
The method selection is shown below under the API drop-down list:
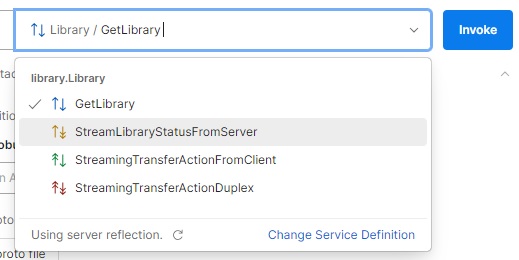
We will use the same message parameter we used for the unary call.
To execute the server stream RPC method call, click the Invoke button.
Once clicked, we will observe every 30 seconds a library status record in JSON format is returned in the Responses tab:
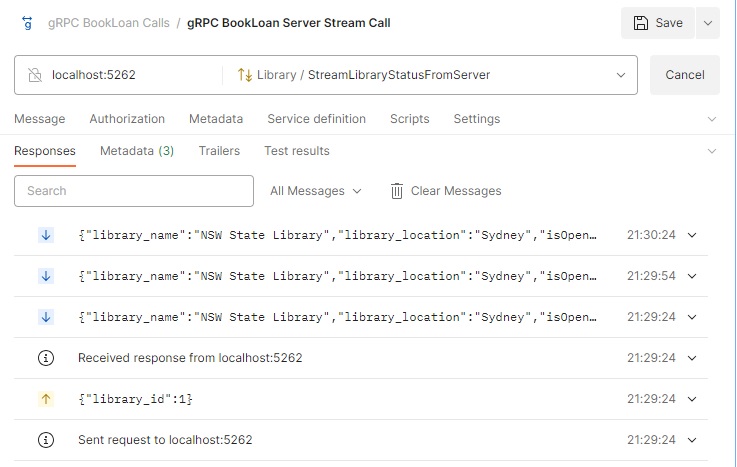
Notice that when we expand any of the received responses, the details of the response in JSON format will display with each property in the response.
In addition, we can cancel the server stream RPC call on demand by clicking the Cancel button as shown below:
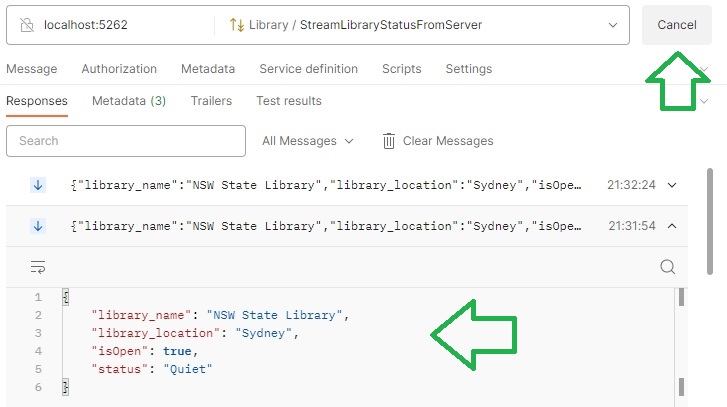
When we click on the cancel button, this triggers the cancellation token, that as I mentioned in the previous post, will cancel the task.
After cancellation, you will see a status code of CANCELLED show to the right of the tabs and the Operation cancelled message as the latest message under the responses log:
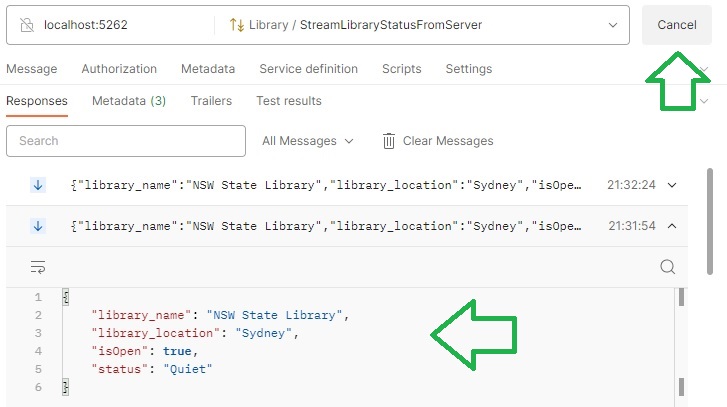
When we click on the cancel button, this triggers the cancellation token, that as I mentioned in the previous post, will cancel the task.
After cancellation, you will see a status code of CANCELLED show to the right of the tabs and the Operation cancelled message as the latest message under the responses log:
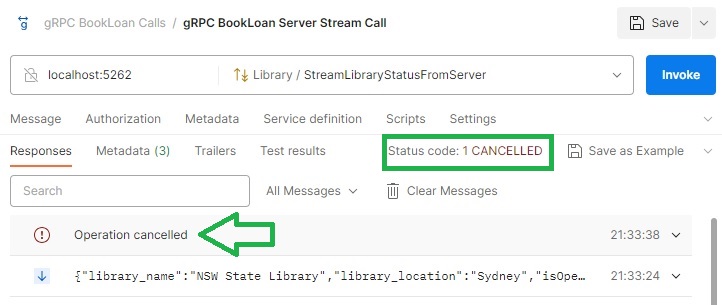
In addition, observing the debug console within the running gRPC .NET Core Server will show a Fail message and a TaskCanceledException exception message:
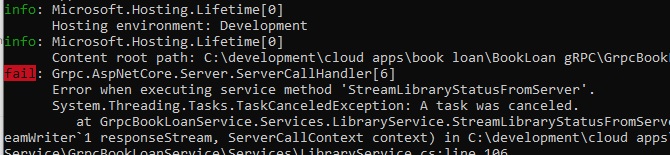
In the next section, I will show how we test RPC Client Stream calls.
Testing an RPC Client Stream Call
In this section, I will now show how to test a client stream RPC call.
In the API drop-down list, I will select the server stream RPC call, StreamingTransferActionFromClient.
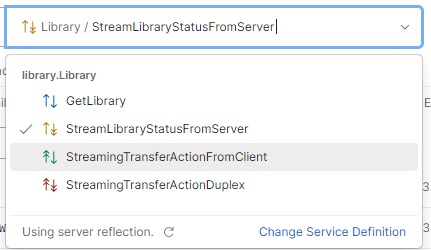
Before I enter any messages, I will need to determine the format of the messages for this request I refer to the Protobuf IDL file, which shows the client stream message format as:
message LibraryBookTransferRequest
{
int32 library_id = 1;
string book_id = 2;
string transfer_action = 3;
google.protobuf.Timestamp date_action = 4;
}
I then select the Message tab and enter the parameters:
{
"library_id": 1,
"book_id": "1",
"transfer_action": "Loan",
"date_action": {"seconds": 1576800000, "nanos": 1000 }
}
Note: the date time parameter requires the seconds and nanos properties. I have set the date to be 50 years past 1970-01-01 (24*60*60*365*50 seconds) and the nanoseconds to 1000.
I then click Invoke to start the request.
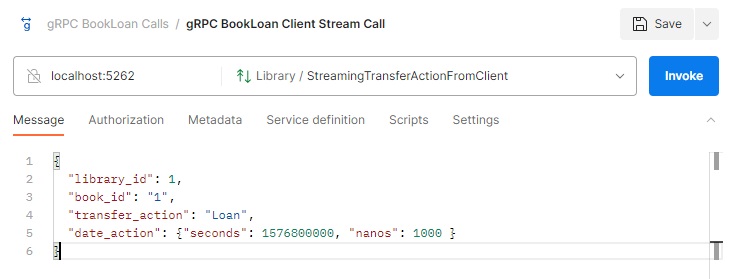
At this point we have not yet submitted the first client message to the server stream.
To submit the first message, click on the Send action.
To send another message in the same client message stream, enter another set of parameters:
{
"library_id": 2,
"book_id": "3",
"transfer_action": "Loan",
"date_action": {"seconds": 1576886400, "nanos": 1000 }
}
Click on the Send action.
Two messages have been submitted to the server.
To end the stream of client messages, click on the End Streaming action.
As we did for the server streaming RPC call, we could have also cancelled the call by clicking on the Cancel action.
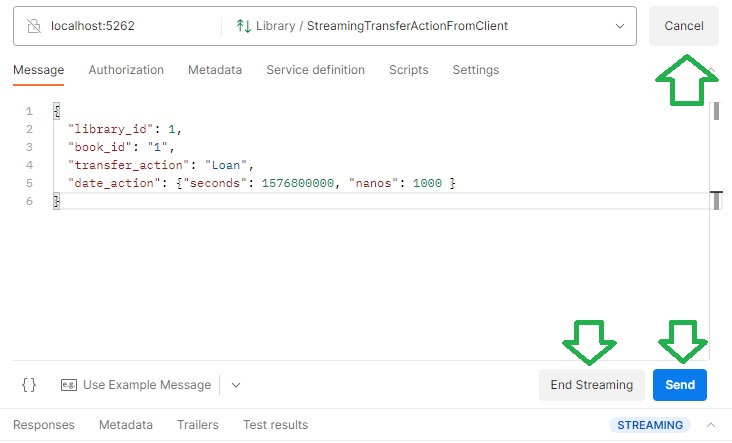
Following the completion of the client stream, the server will send a response reply back to the Postman client:
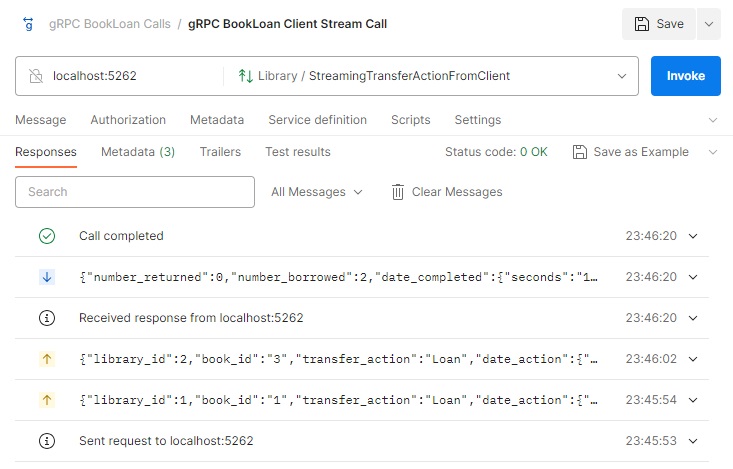
The response will be a JSON message with the total number of loans and return client request messages within the 10 second interval from the first received message.
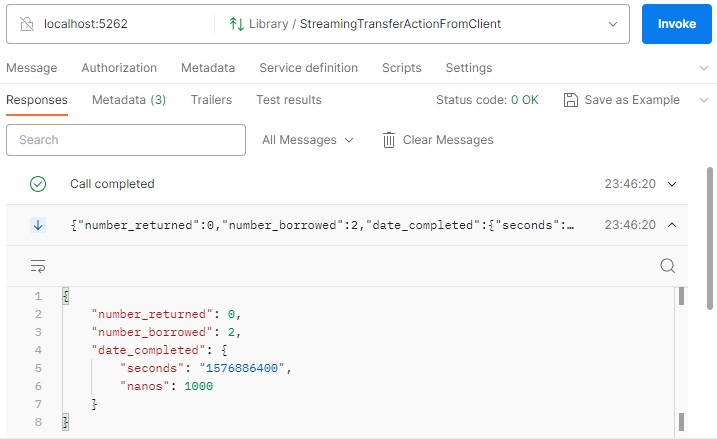
Note that if we had submitted messages with the following date/time formats for the date time field:
“date_action”: “02-09-2023 06:15:00 PM”
OR
“date_action”: { “2023-09-02T06:15:00Z”}
Then we would have got an error when trying to Invoke the call.
In the next section, I will show how to test an RPC Duplex Streaming Call.
Testing an RPC Duplex Stream Call
In this section, I will show how to test a duplex stream RPC call.
In the API method drop-down list, I will select the server stream RPC call, StreamingTransferActionDuplex.
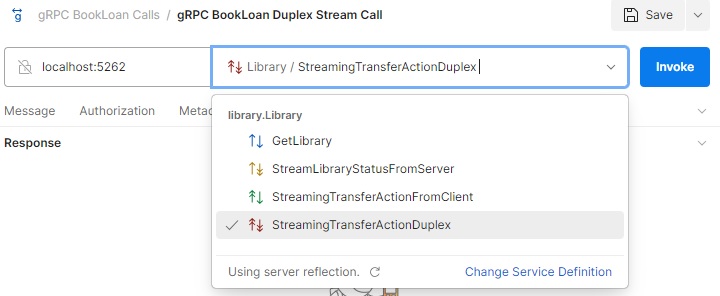
With this call, the client messages I will be sending to the server will be in the same message format as the server response messages. These will be in the following message format:
message LibraryBookTransferRequest
{
int32 library_id = 1;
string book_id = 2;
string transfer_action = 3;
google.protobuf.Timestamp date_action = 4;
}
For the test, I will send the following three messages in between the 10 second intervals that the server will be sending random messages back to the Postman client:
{
"library_id": 1,
"book_id": "1",
"transfer_action": "Loan",
"date_action": {"seconds": 1576800000, "nanos": 1000 }
}
{
"library_id": 2,
"book_id": "4",
"transfer_action": "Loan",
"date_action": {"seconds": 1576800000, "nanos": 1000 }
}
{
"library_id": 3,
"book_id": "2",
"transfer_action": "Return",
"date_action": {"seconds": 1576800000, "nanos": 1000 }
}
After dispatching the three messages within a 30 second interval, I receive some randomly generated messages from the RPC server. On the server debug console, I have tracked the number of loan and return messages:
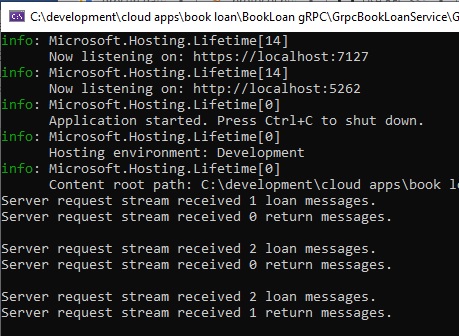
After ending the client stream, the server stream ends and the response log in Postman shows both messages sent from the client (indicated by the green arrows) and messages received from the server (indicated by the blue arrows):
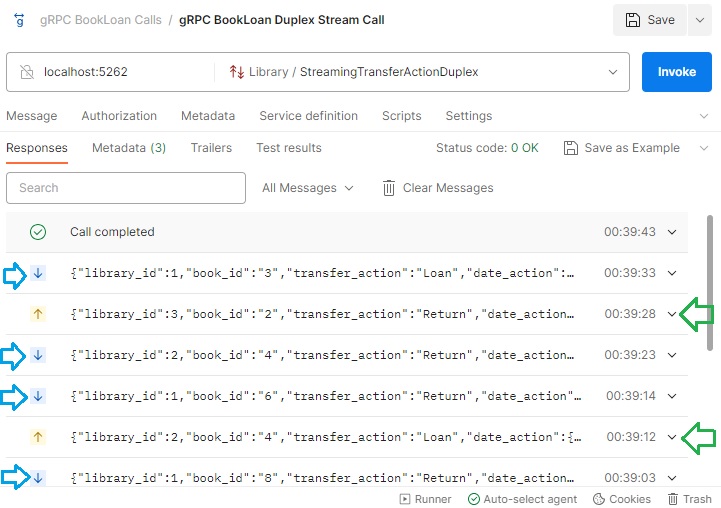
Below is a typical random message received from the RPC server with the current date and time:
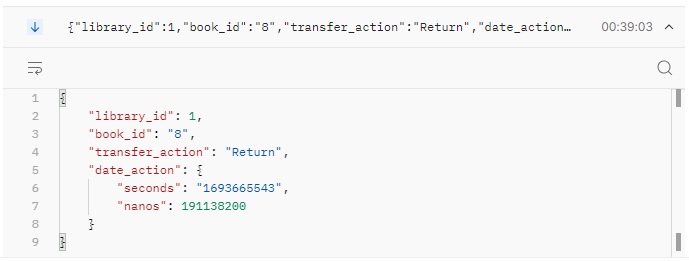
In this post we have seen how to test the four different types of RPC call types from Postman to a running gRPC .NET Core server.
That is all for today’s post.
I hope you have found this post useful and informative.
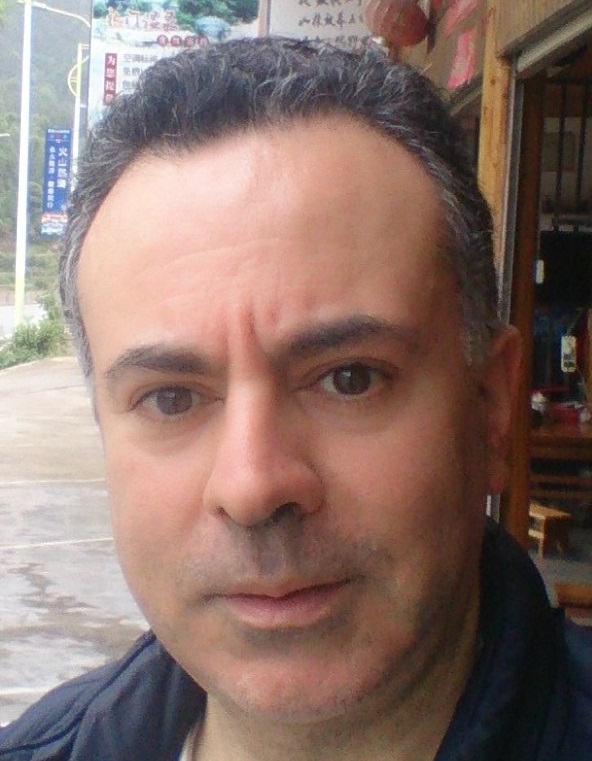
Andrew Halil is a blogger, author and software developer with expertise of many areas in the information technology industry including full-stack web and native cloud based development, test driven development and Devops.